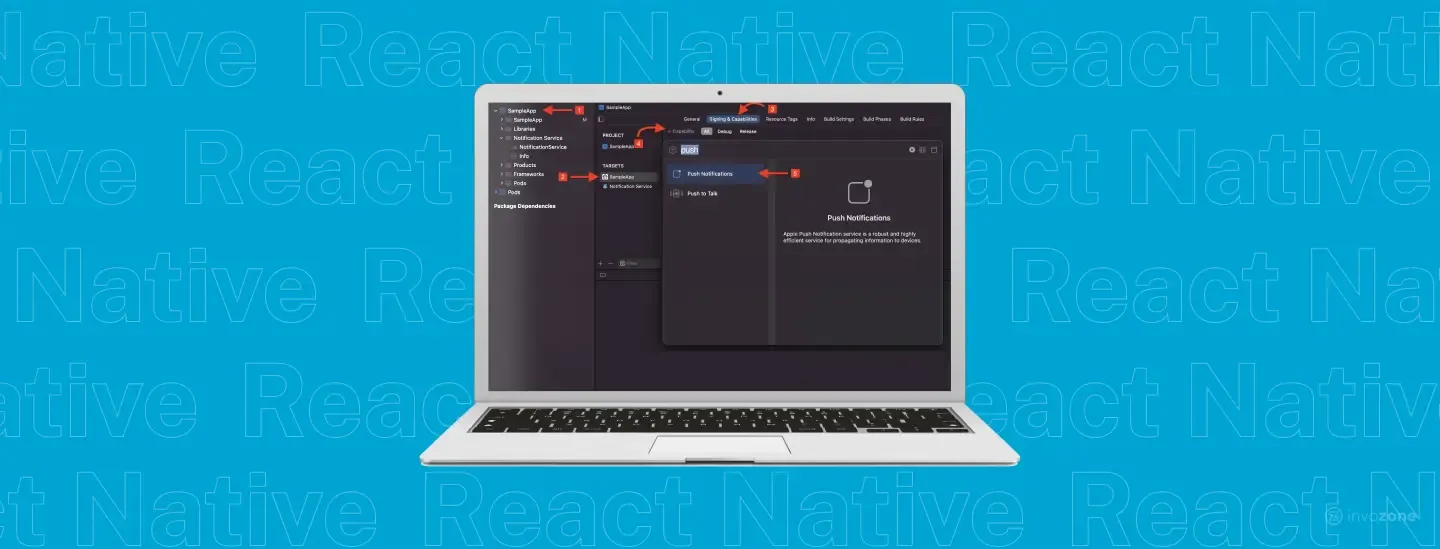
How to Implement Push Notifications in a React Native Application
This blog guides you through integrating push notifications in a React Native app using Firebase Cloud Messaging (FCM). Learn how to set up Firebase, configure Android and iOS, handle notifications, and test your setup for enhanced user engagement.
Published On: 28 August, 2024
4 min read
Table of Contents
- After Reading This Blog Post, You’ll Know:
- Why Use Push Notifications?
- Prerequisites
- Step 1: Set Up Firebase Project
- Step 2: Install Required Libraries
- Step 3: Configure Android
- Step 4: Changing the Notification Icon For Android
- Step 4: Configure iOS
- Step 5: Request Notification Permissions
- Step 6: Handle Incoming Messages
- Step 7: Test Push Notifications
- Step 8: Add Notification Listeners
- Step 9: Send Push Notifications From FCM
- Conclusion
Push notifications are a major leap forward in mobile app development, offering a direct line to engage users and keep them informed about important updates.
In this blog, we'll walk you through the process of integrating push notifications into your React Native application using Firebase Cloud Messaging (FCM). Whether you're building a new app or enhancing an existing one, mastering push notifications will enable you to provide timely and relevant information to your users, boosting engagement and retention.
Follow our step-by-step guide to seamlessly add this powerful feature to your app.
After Reading This Blog Post, You’ll Know:
- Firebase Project Setup: How to create and configure a Firebase project for push notifications.
- Library Installation: Installing and linking necessary libraries for React Native.
- Android Configuration: Setting up Firebase messaging for Android devices, including notification icons.
- iOS Configuration: Integrating Firebase messaging with iOS, including adding necessary files and pods.
- Permission Requests: How to request notification permissions from users.
- Handling Incoming Messages: Implementing listeners to manage incoming notifications.
- Testing Notifications: Obtaining FCM tokens and sending test notifications.
- Advanced Notification Handling: Adding listeners for app state changes to handle notifications effectively.
Why Use Push Notifications?
Push notifications are a crucial tool for keeping users engaged and informed in today’s mobile-centric world. By sending timely and relevant messages directly to a user’s device, push notifications can significantly enhance the user experience and drive app retention. Here’s why integrating push notifications into your React Native application can be a game-changer:
- Boost User Engagement: Push notifications can prompt users to return to your app by delivering personalized messages, updates, and reminders. This direct communication helps keep your app top-of-mind, encouraging users to engage more frequently.
- Increase Retention Rates: Regularly sending notifications about new features, updates, or important information helps maintain user interest and loyalty. By reminding users of your app’s value and relevance, you can improve long-term retention and reduce churn.
- Drive User Action: Notifications can be used to drive specific actions, such as completing a purchase, participating in a promotion, or reading new content. By crafting compelling messages and leveraging targeted notifications, you can influence user behavior and achieve your business goals.
- Provide Real-Time Updates: Whether it’s a breaking news alert, a chat message, or a live sports score, push notifications enable you to deliver real-time updates to users. This immediacy ensures that users receive important information as soon as it’s available, enhancing the timeliness and relevance of your communication.
- Personalize User Experience: With push notifications, you can tailor messages based on user preferences, behaviors, and location. This personalization increases the effectiveness of your notifications, making them more relevant and engaging to each individual user.
- Enhance User Experience: Well-timed and relevant notifications can improve the overall user experience by keeping users informed and engaged without requiring them to actively check the app. This convenience can lead to higher satisfaction and a more positive perception of your app.
- Promote New Features and Content: Use notifications to announce new features, content updates, or upcoming events. This proactive approach helps ensure that users are aware of what’s new and encourages them to explore the latest offerings.
- Encourage User Feedback: Notifications can be used to solicit feedback, encourage reviews, or conduct surveys. By reaching out to users directly, you can gather valuable insights and improve your app based on user input.
Prerequisites
Before we begin, make sure you have the following:
- A React Native project set up.
- Node.js and npm are installed on your development machine.
- An active Firebase account.
Step 1: Set Up Firebase Project
- Go to the Firebase Console.
- Click on Add Project and follow the instructions to create a new Firebase project.
- Once your project is created, navigate to Project Settings.
- Under the General tab, scroll down to Your Apps and click on the Add App button.
- Choose your platform (iOS or Android) and follow the steps to register your app.
Step 2: Install Required Libraries
Install the following libraries in your React Native project:
npm install @react-native-firebase/app
npm install @react-native-firebase/messaging
//For yarn
yarn add @react-native-firebase/app
yarn add @react-native-firebase/messaging
Link the libraries to your project (for older versions of React Native):
react-native link @react-native-firebase/app
react-native link @react-native-firebase/messaging
For React Native 0.60 and above, auto-linking should handle the linking of these libraries.
Step 3: Configure Android
- Open your android/app/build.gradle file and add the following line to the dependencies section:
implementation 'com.google.firebase:firebase-messaging:23.0.0'
- In your android/build.gradle file, ensure you have the Google services classpath:
classpath 'com.google.gms:google-services:4.3.3'
- Apply the Google services plugin at the bottom of the android/app/build.gradle file:
apply plugin: 'com.google.gms.google-services'
- Add the google-services.json file you downloaded from the Firebase Console to the android/app/ directory.
Step 4: Changing the Notification Icon For Android
Open android/app/src/main/AndroidManifest.xml and add the following within the <application> tag:
<application>
<!-- ... -->
<meta-data
android:name="com.google.firebase.messaging.default_notification_icon"
android:resource="@drawable/ic_notification_icon" />
<!-- ... -->
</application>
Step 4: Configure iOS
- Navigate to your project in Xcode.
- Open ios/YourProjectName/ and add the GoogleService-Info.plist file downloaded from the Firebase Console.
- In your ios/Podfile, add the following line:
pod 'Firebase/Messaging'
-
Run pod install in the ios directory to install the Firebase Messaging pod.
Step 5: Request Notification Permissions
In your React Native app, request permission to send notifications:
import messaging from '@react-native-firebase/messaging';
async function requestUserPermission() {
const authStatus = await messaging().requestPermission();
const enabled =
authStatus === messaging.AuthorizationStatus.AUTHORIZED ||
authStatus === messaging.AuthorizationStatus.PROVISIONAL;
if (enabled) {
console.log('Authorization status:', authStatus);
}
}
useEffect(() => {
requestUserPermission();
}, []);
Step 6: Handle Incoming Messages
To handle incoming messages, set up a listener in your app:
useEffect(() => {
const unsubscribe = messaging().onMessage(async remoteMessage => {
Alert.alert('A new FCM message arrived!', JSON.stringify(remoteMessage));
});
return unsubscribe;
}, []);
Step 7: Test Push Notifications
-
Obtain the FCM token for your device:
useEffect(() => {
messaging()
.getToken()
.then(token => {
console.log('FCM Token:', token);
});
}, []);
- Use the Firebase Console to send a test notification to your app using the obtained FCM token.
Step 8: Add Notification Listeners
-
Check the app state
const notificationListener = () => {
messaging().onNotificationOpenedApp(remoteMessage => {
console.log(
'Notification caused app to open from background state:',
remoteMessage.notification,
);
});
messaging()
.getInitialNotification()
.then(remoteMessage => {
if (remoteMessage) {
console.log(
'Notification caused app to open from quit state:',
remoteMessage.notification,
);
}
})
.catch(error => console.log('failed', error));
};
useEffect(()=>{
notificationListener()
},[])
Step 9: Send Push Notifications From FCM
- Go to the FCM dashboard to send messages. In the Engage>Cloud Messaging section, click on compose notification.
- Enter the notification values and press send text message. The notification will be received on your phone.
Congratulations! You've successfully integrated push notifications into your React Native app. This feature will help you keep your users engaged and informed.
If you're looking for professional assistance with developing or enhancing your mobile application, check out our hybrid mobile app development services to see how we can help bring your project to life.
Conclusion
Integrating push notifications into your React Native application is a powerful way to enhance user engagement, boost retention, and provide timely updates directly to your users. By following this step-by-step guide, you’ve unlocked a key feature that can transform how your app communicates with its audience.
From setting up Firebase and configuring both Android and iOS to handling notifications and testing them effectively, you now have the tools to implement this essential functionality.
Push notifications not only keep users informed about new content and updates but also drive meaningful interactions and foster a more dynamic user experience. With personalized and well-timed messages, you can maintain user interest, encourage action, and ultimately create a more compelling and engaging app.
As you move forward, continue to refine your notification strategies and explore advanced features to maximize their impact. Whether it’s optimizing notification delivery or experimenting with targeted messaging, there’s always room to innovate and improve.
Congratulations on mastering push notifications in React Native! If you need further assistance or want to take your app development to the next level, consider reaching out for expert help. We’re here to support you in bringing your mobile app vision to life with our comprehensive development services.
Happy coding, and here’s to the success of your enhanced app experience!
Don’t Have Time To Read Now? Download It For Later.
Table of Contents
- After Reading This Blog Post, You’ll Know:
- Why Use Push Notifications?
- Prerequisites
- Step 1: Set Up Firebase Project
- Step 2: Install Required Libraries
- Step 3: Configure Android
- Step 4: Changing the Notification Icon For Android
- Step 4: Configure iOS
- Step 5: Request Notification Permissions
- Step 6: Handle Incoming Messages
- Step 7: Test Push Notifications
- Step 8: Add Notification Listeners
- Step 9: Send Push Notifications From FCM
- Conclusion
Push notifications are a major leap forward in mobile app development, offering a direct line to engage users and keep them informed about important updates.
In this blog, we'll walk you through the process of integrating push notifications into your React Native application using Firebase Cloud Messaging (FCM). Whether you're building a new app or enhancing an existing one, mastering push notifications will enable you to provide timely and relevant information to your users, boosting engagement and retention.
Follow our step-by-step guide to seamlessly add this powerful feature to your app.
After Reading This Blog Post, You’ll Know:
- Firebase Project Setup: How to create and configure a Firebase project for push notifications.
- Library Installation: Installing and linking necessary libraries for React Native.
- Android Configuration: Setting up Firebase messaging for Android devices, including notification icons.
- iOS Configuration: Integrating Firebase messaging with iOS, including adding necessary files and pods.
- Permission Requests: How to request notification permissions from users.
- Handling Incoming Messages: Implementing listeners to manage incoming notifications.
- Testing Notifications: Obtaining FCM tokens and sending test notifications.
- Advanced Notification Handling: Adding listeners for app state changes to handle notifications effectively.
Why Use Push Notifications?
Push notifications are a crucial tool for keeping users engaged and informed in today’s mobile-centric world. By sending timely and relevant messages directly to a user’s device, push notifications can significantly enhance the user experience and drive app retention. Here’s why integrating push notifications into your React Native application can be a game-changer:
- Boost User Engagement: Push notifications can prompt users to return to your app by delivering personalized messages, updates, and reminders. This direct communication helps keep your app top-of-mind, encouraging users to engage more frequently.
- Increase Retention Rates: Regularly sending notifications about new features, updates, or important information helps maintain user interest and loyalty. By reminding users of your app’s value and relevance, you can improve long-term retention and reduce churn.
- Drive User Action: Notifications can be used to drive specific actions, such as completing a purchase, participating in a promotion, or reading new content. By crafting compelling messages and leveraging targeted notifications, you can influence user behavior and achieve your business goals.
- Provide Real-Time Updates: Whether it’s a breaking news alert, a chat message, or a live sports score, push notifications enable you to deliver real-time updates to users. This immediacy ensures that users receive important information as soon as it’s available, enhancing the timeliness and relevance of your communication.
- Personalize User Experience: With push notifications, you can tailor messages based on user preferences, behaviors, and location. This personalization increases the effectiveness of your notifications, making them more relevant and engaging to each individual user.
- Enhance User Experience: Well-timed and relevant notifications can improve the overall user experience by keeping users informed and engaged without requiring them to actively check the app. This convenience can lead to higher satisfaction and a more positive perception of your app.
- Promote New Features and Content: Use notifications to announce new features, content updates, or upcoming events. This proactive approach helps ensure that users are aware of what’s new and encourages them to explore the latest offerings.
- Encourage User Feedback: Notifications can be used to solicit feedback, encourage reviews, or conduct surveys. By reaching out to users directly, you can gather valuable insights and improve your app based on user input.
Prerequisites
Before we begin, make sure you have the following:
- A React Native project set up.
- Node.js and npm are installed on your development machine.
- An active Firebase account.
Step 1: Set Up Firebase Project
- Go to the Firebase Console.
- Click on Add Project and follow the instructions to create a new Firebase project.
- Once your project is created, navigate to Project Settings.
- Under the General tab, scroll down to Your Apps and click on the Add App button.
- Choose your platform (iOS or Android) and follow the steps to register your app.
Step 2: Install Required Libraries
Install the following libraries in your React Native project:
npm install @react-native-firebase/app
npm install @react-native-firebase/messaging
//For yarn
yarn add @react-native-firebase/app
yarn add @react-native-firebase/messaging
Link the libraries to your project (for older versions of React Native):
react-native link @react-native-firebase/app
react-native link @react-native-firebase/messaging
For React Native 0.60 and above, auto-linking should handle the linking of these libraries.
Step 3: Configure Android
- Open your android/app/build.gradle file and add the following line to the dependencies section:
implementation 'com.google.firebase:firebase-messaging:23.0.0'
- In your android/build.gradle file, ensure you have the Google services classpath:
classpath 'com.google.gms:google-services:4.3.3'
- Apply the Google services plugin at the bottom of the android/app/build.gradle file:
apply plugin: 'com.google.gms.google-services'
- Add the google-services.json file you downloaded from the Firebase Console to the android/app/ directory.
Step 4: Changing the Notification Icon For Android
Open android/app/src/main/AndroidManifest.xml and add the following within the <application> tag:
<application>
<!-- ... -->
<meta-data
android:name="com.google.firebase.messaging.default_notification_icon"
android:resource="@drawable/ic_notification_icon" />
<!-- ... -->
</application>
Step 4: Configure iOS
- Navigate to your project in Xcode.
- Open ios/YourProjectName/ and add the GoogleService-Info.plist file downloaded from the Firebase Console.
- In your ios/Podfile, add the following line:
pod 'Firebase/Messaging'
-
Run pod install in the ios directory to install the Firebase Messaging pod.
Step 5: Request Notification Permissions
In your React Native app, request permission to send notifications:
import messaging from '@react-native-firebase/messaging';
async function requestUserPermission() {
const authStatus = await messaging().requestPermission();
const enabled =
authStatus === messaging.AuthorizationStatus.AUTHORIZED ||
authStatus === messaging.AuthorizationStatus.PROVISIONAL;
if (enabled) {
console.log('Authorization status:', authStatus);
}
}
useEffect(() => {
requestUserPermission();
}, []);
Step 6: Handle Incoming Messages
To handle incoming messages, set up a listener in your app:
useEffect(() => {
const unsubscribe = messaging().onMessage(async remoteMessage => {
Alert.alert('A new FCM message arrived!', JSON.stringify(remoteMessage));
});
return unsubscribe;
}, []);
Step 7: Test Push Notifications
-
Obtain the FCM token for your device:
useEffect(() => {
messaging()
.getToken()
.then(token => {
console.log('FCM Token:', token);
});
}, []);
- Use the Firebase Console to send a test notification to your app using the obtained FCM token.
Step 8: Add Notification Listeners
-
Check the app state
const notificationListener = () => {
messaging().onNotificationOpenedApp(remoteMessage => {
console.log(
'Notification caused app to open from background state:',
remoteMessage.notification,
);
});
messaging()
.getInitialNotification()
.then(remoteMessage => {
if (remoteMessage) {
console.log(
'Notification caused app to open from quit state:',
remoteMessage.notification,
);
}
})
.catch(error => console.log('failed', error));
};
useEffect(()=>{
notificationListener()
},[])
Step 9: Send Push Notifications From FCM
- Go to the FCM dashboard to send messages. In the Engage>Cloud Messaging section, click on compose notification.
- Enter the notification values and press send text message. The notification will be received on your phone.
Congratulations! You've successfully integrated push notifications into your React Native app. This feature will help you keep your users engaged and informed.
If you're looking for professional assistance with developing or enhancing your mobile application, check out our hybrid mobile app development services to see how we can help bring your project to life.
Conclusion
Integrating push notifications into your React Native application is a powerful way to enhance user engagement, boost retention, and provide timely updates directly to your users. By following this step-by-step guide, you’ve unlocked a key feature that can transform how your app communicates with its audience.
From setting up Firebase and configuring both Android and iOS to handling notifications and testing them effectively, you now have the tools to implement this essential functionality.
Push notifications not only keep users informed about new content and updates but also drive meaningful interactions and foster a more dynamic user experience. With personalized and well-timed messages, you can maintain user interest, encourage action, and ultimately create a more compelling and engaging app.
As you move forward, continue to refine your notification strategies and explore advanced features to maximize their impact. Whether it’s optimizing notification delivery or experimenting with targeted messaging, there’s always room to innovate and improve.
Congratulations on mastering push notifications in React Native! If you need further assistance or want to take your app development to the next level, consider reaching out for expert help. We’re here to support you in bringing your mobile app vision to life with our comprehensive development services.
Happy coding, and here’s to the success of your enhanced app experience!
Share to:
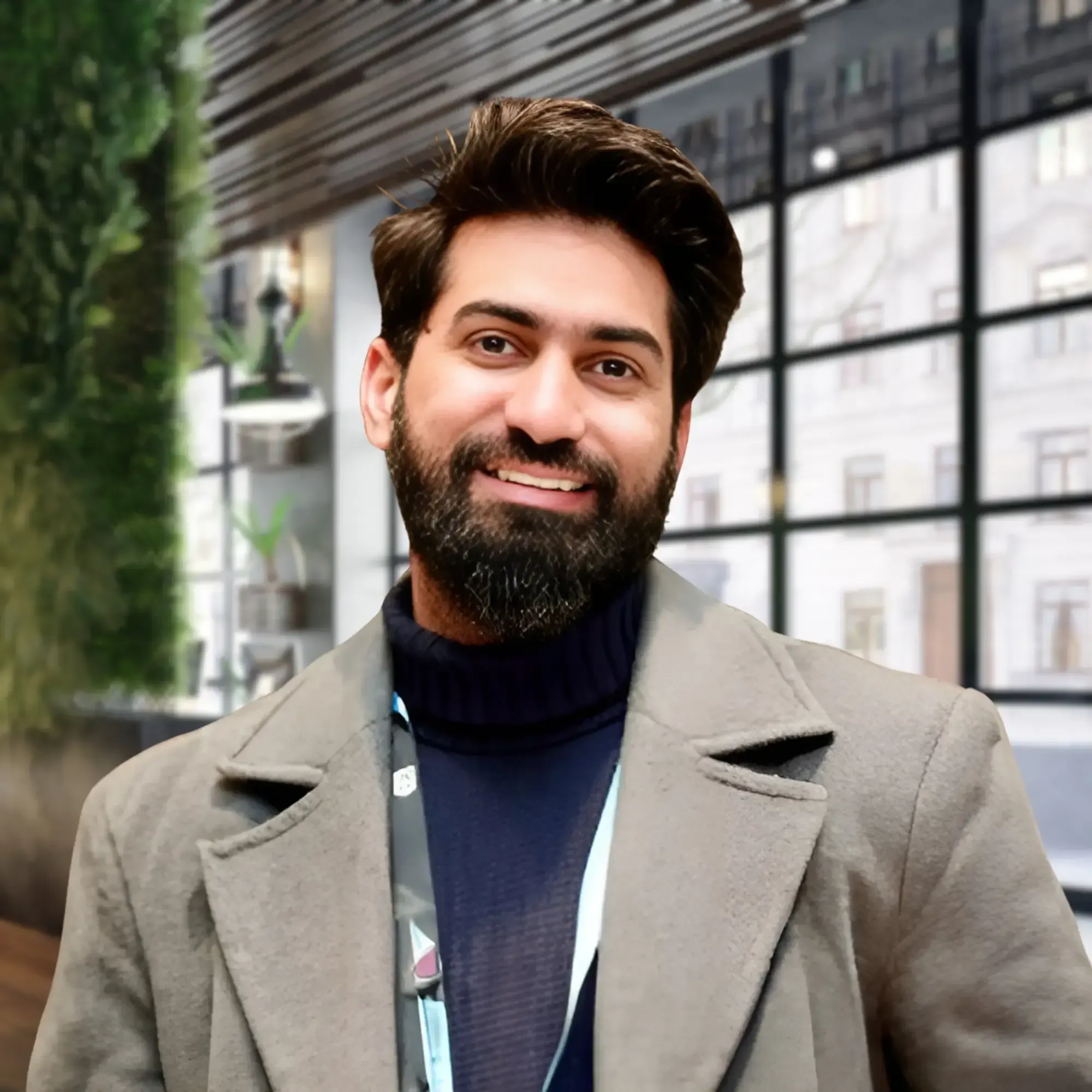
Written By:
Furqan AzizFurqan Aziz is CEO & Founder of InvoZone. He is a tech enthusiast by heart with 10+ years ... Know more
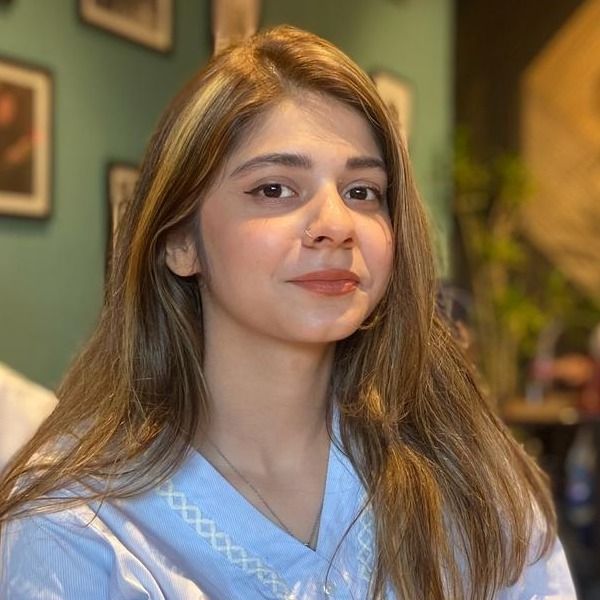
Contributed By:
Senior Content Lead
Get Help From Experts At InvoZone In This Domain