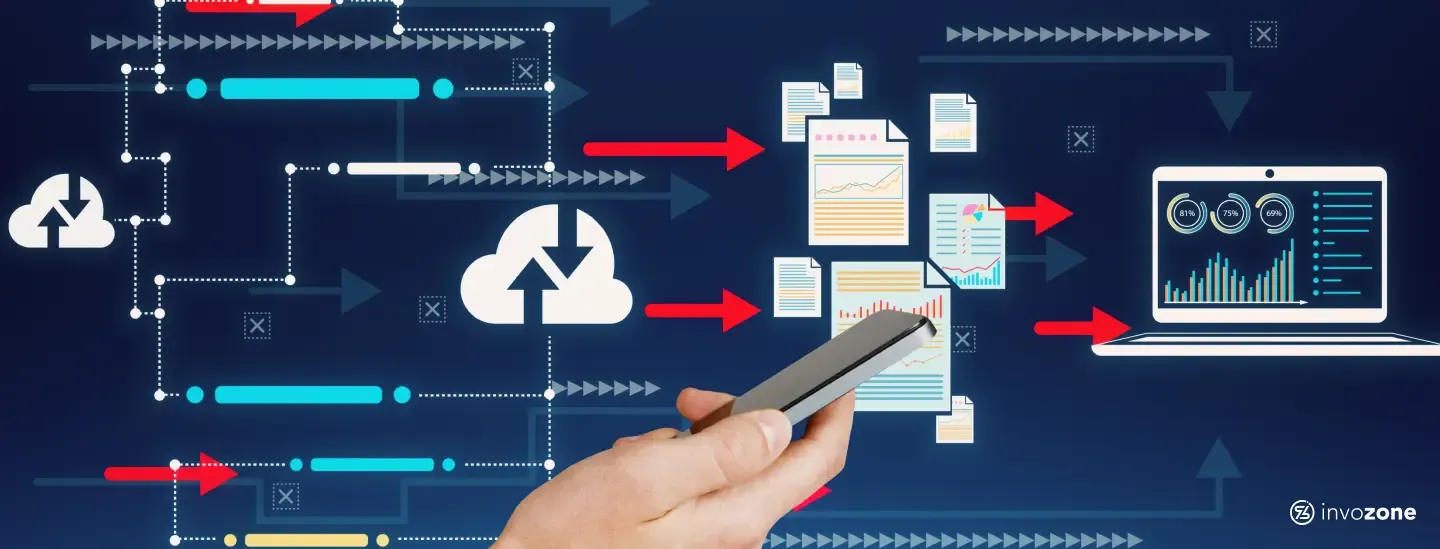
How to Deploy a Microservices Application onto Kubernetes with Auto-Scaling
This blog covers deploying microservices on Kubernetes, including containerization with Docker, setting up Kubernetes deployments, enabling auto-scaling, and using NGINX Ingress for traffic management. Learn how Kubernetes’ features streamline scaling and improve application performance.
Published On: 28 August, 2024
4 min read
Table of Contents
In the ever-evolving world of software development, microservices have emerged as a powerful architectural approach, allowing teams to build and scale applications with unprecedented flexibility.
This method breaks down complex applications into smaller, manageable services that can be developed, deployed, and scaled independently. Kubernetes, a leading open-source container orchestration platform, complements this architecture perfectly by providing robust tools for managing these services efficiently.
Kubernetes simplifies the deployment, scaling, and operation of containerized applications, making it an ideal choice for modern software environments. It offers automated scaling, load balancing, and recovery from failures, which are crucial for maintaining the performance and reliability of applications in production.
We'll cover everything from containerizing your microservices to configuring Kubernetes deployments and services, and finally setting up auto-scaling to ensure your application can handle varying loads with ease.
Why Kubernetes is Ideal for Microservices
Kubernetes is renowned for its ability to manage complex, distributed applications. For microservices architecture, Kubernetes provides:
- Automated Scaling: Dynamically adjust the number of running instances based on real-time demand.
- Load Balancing: Distribute incoming traffic across multiple instances to ensure even load and high availability.
- Self-Healing: Automatically replace failed containers and ensure the application remains healthy.
- Service Discovery: Simplify communication between services through internal DNS and networking.
These features make Kubernetes an essential tool for deploying and managing microservices in a way that is both efficient and resilient.
What You Need Before You Start
To get started with deploying your microservices on Kubernetes, ensure you have the following prerequisites in place:
- Basic Knowledge: A foundational understanding of Docker and Kubernetes will be essential as we’ll be working with container images and Kubernetes configurations.
- Kubernetes Cluster: Access to a Kubernetes cluster is required. This could be a local setup like Minikube for development or a managed cloud service such as Google Kubernetes Engine (GKE), Amazon EKS, or Azure AKS for production.
- Docker: Docker should be installed on your development machine to build and manage container images.
With these elements in place, you’re ready to embark on the journey of deploying your microservices on Kubernetes and harnessing its power to achieve efficient and scalable application management.
Why Use Kubernetes for Microservices?
Kubernetes provides robust tools to manage and scale microservices efficiently. It allows for easy scaling, load balancing, and automatic recovery from failures, making it an ideal choice for deploying modern applications.
Prerequisites
Before you start, ensure you have the following:
- Basic understanding of Docker and Kubernetes.
- Kubernetes cluster set up. You can use a local setup like Minikube or a cloud provider like Google Kubernetes Engine (GKE), Amazon EKS, or Azure AKS.
- Docker installed on your development machine.
Step 1: Containerize Your Microservices
-
Create Dockerfiles for each microservice. Here’s an example of a simple Node.js microservice Dockerfile:
You can create multiple microservices with different ports, for example:
- Frontend microservice: Expose port 8080
- Backend microservice: Expose port 3000
- Admin-panel microservice: Expose port 5000
Here are some examples of Dockerfiles for each microservice:
-
Frontend microservice:
# Use the official Node.js image.
FROM node:14
# Set the working directory.
WORKDIR /usr/src/app
# Copy package.json and install dependencies.
COPY package*.json ./
RUN npm install
# Copy the rest of the application code.
COPY . .
# Expose the port your app runs on.
EXPOSE 8080
# Start the application.
CMD ["node", "app.js"]
-
Backend microservice:
# Use the official Node.js image.
FROM node:20
# Set the working directory.
WORKDIR /usr/src/app
# Copy package.json and install dependencies.
COPY package*.json ./
RUN npm install
# Copy the rest of the application code.
COPY . .
# Expose the port your app runs on.
EXPOSE 3000
# Start the application.
CMD ["node", "app.js"]
Build Docker images for each service like:
docker build -t my-microservice:latest
Push Docker images to a container registry like Docker Hub or Google Container Registry (GCR):
docker push myusername/my-microservice:latest
Step 2: Deploy Microservices on Kubernetes
-
Create Kubernetes Deployment and Service files for each microservice. Here’s an example for a deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-microservice
spec:
replicas: 2
selector:
matchLabels:
app: my-microservice
template:
metadata:
labels:
app: my-microservice
spec:
containers:
- name: my-microservice
image: myusername/my-microservice:latest
ports:
- containerPort: 8080
-
And a service file for each microservice:
apiVersion: v1
kind: Service
metadata:
name: my-microservice
spec:
type: ClusterIP
selector:
app: my-microservice
ports:
- port: 8080
targetPort: 8080
-
Apply these configurations to your Kubernetes cluster:
kubectl apply -f my-microservice-deployment.yaml
kubectl apply -f my-microservice-service.yaml
Step 3: Enable Auto-Scaling
-
Set up the Horizontal Pod Autoscaler (HPA) to automatically scale the number of pods in your deployment based on CPU usage:
kubectl autoscale deployment my-microservice --cpu-percent=50 --min=1 --max=10
Monitor the HPA using:
kubectl get hpa
This will show you the current CPU usage and the desired number of replicas.
Step 4: Now expose the services via NGINX-Ingress
-
Install the nginx-ingress service via helm chart from which we can expose application for the access from browser:
Step 1: Add the NGINX Ingress Helm repository
Run the following command to add the NGINX Ingress Helm repository:
helm repo add nginx-stable https://helm.nginx.com/stable
Step 2: Update the Helm repository
Run the following command to update the Helm repository:
helm repo update
Step 3: Install the NGINX Ingress Controller
Run the following command to install the NGINX Ingress Controller:
helm install nginx-ingress nginx-stable/nginx-ingress
Now create file named “ingress.yaml” to apply in the kubernete:
apiVersion: networking.k8s.io/v1beta1
kind: Ingress
metadata:
name: netflix-ingress
spec:
tls:
- hosts:
- frontend.example.com
secretName: frontend-tls
- hosts:
- backend.example.com
secretName: backed-tls
- hosts:
- admin.example.com
secretName: admin-tls
rules:
- host: frontend.example.com
http:
paths:
- path: /
backend:
serviceName: frontend
servicePort: 8080
- host: frontend.example.com
http:
paths:
- path: /
backend:
serviceName: backend
servicePort: 3000
- host: backend.example.com
http:
paths:
- path: /
backend:
serviceName: admin
servicePort: 5000
Install the SSL certificates:
You'll need to create a Kubernetes secret for each SSL certificate, using the following command:
kubectl create secret tls frontend-tls --key /certs/frontend.key --cert /certs/frontend.crt
kubectl create secret tls backend-tls --key /certs/backend.key --cert /certs/backend.crt
kubectl create secret tls admin-tls --key /certs/admin.key --cert /certs/admin.crt
Finally apply the ingress.yaml
kubectl apply -f ingress.yaml
Step 4: Test and Monitor
- Test your deployment by simulating load using tools like Apache JMeter or Locust to ensure that the auto-scaling works as expected.
- Monitor your cluster with the Kubernetes dashboard or other monitoring tools like Prometheus and Grafana to get insights into the performance and health of your services.
Conclusion
By now, you’ve seen how Kubernetes can transform the way you deploy and manage your microservices. From containerizing your applications to setting up auto-scaling and traffic management, Kubernetes offers the tools you need to handle modern workloads with ease.
Implementing these practices will not only enhance your application's performance but also streamline your deployment processes, making it easier to scale and adapt to changing demands.
Ready to leverage Kubernetes for your next project? Dive into the details, experiment with the configurations, and watch your applications thrive. For more tips, resources, and expert guidance, stay tuned to our blog or contact us for personalized support. Happy deploying!
If you need professional assistance with cloud and DevOps solutions, explore our cloud and DevOps services to see how we can help you streamline your operations and maximize performance.
Don’t Have Time To Read Now? Download It For Later.
Table of Contents
In the ever-evolving world of software development, microservices have emerged as a powerful architectural approach, allowing teams to build and scale applications with unprecedented flexibility.
This method breaks down complex applications into smaller, manageable services that can be developed, deployed, and scaled independently. Kubernetes, a leading open-source container orchestration platform, complements this architecture perfectly by providing robust tools for managing these services efficiently.
Kubernetes simplifies the deployment, scaling, and operation of containerized applications, making it an ideal choice for modern software environments. It offers automated scaling, load balancing, and recovery from failures, which are crucial for maintaining the performance and reliability of applications in production.
We'll cover everything from containerizing your microservices to configuring Kubernetes deployments and services, and finally setting up auto-scaling to ensure your application can handle varying loads with ease.
Why Kubernetes is Ideal for Microservices
Kubernetes is renowned for its ability to manage complex, distributed applications. For microservices architecture, Kubernetes provides:
- Automated Scaling: Dynamically adjust the number of running instances based on real-time demand.
- Load Balancing: Distribute incoming traffic across multiple instances to ensure even load and high availability.
- Self-Healing: Automatically replace failed containers and ensure the application remains healthy.
- Service Discovery: Simplify communication between services through internal DNS and networking.
These features make Kubernetes an essential tool for deploying and managing microservices in a way that is both efficient and resilient.
What You Need Before You Start
To get started with deploying your microservices on Kubernetes, ensure you have the following prerequisites in place:
- Basic Knowledge: A foundational understanding of Docker and Kubernetes will be essential as we’ll be working with container images and Kubernetes configurations.
- Kubernetes Cluster: Access to a Kubernetes cluster is required. This could be a local setup like Minikube for development or a managed cloud service such as Google Kubernetes Engine (GKE), Amazon EKS, or Azure AKS for production.
- Docker: Docker should be installed on your development machine to build and manage container images.
With these elements in place, you’re ready to embark on the journey of deploying your microservices on Kubernetes and harnessing its power to achieve efficient and scalable application management.
Why Use Kubernetes for Microservices?
Kubernetes provides robust tools to manage and scale microservices efficiently. It allows for easy scaling, load balancing, and automatic recovery from failures, making it an ideal choice for deploying modern applications.
Prerequisites
Before you start, ensure you have the following:
- Basic understanding of Docker and Kubernetes.
- Kubernetes cluster set up. You can use a local setup like Minikube or a cloud provider like Google Kubernetes Engine (GKE), Amazon EKS, or Azure AKS.
- Docker installed on your development machine.
Step 1: Containerize Your Microservices
-
Create Dockerfiles for each microservice. Here’s an example of a simple Node.js microservice Dockerfile:
You can create multiple microservices with different ports, for example:
- Frontend microservice: Expose port 8080
- Backend microservice: Expose port 3000
- Admin-panel microservice: Expose port 5000
Here are some examples of Dockerfiles for each microservice:
-
Frontend microservice:
# Use the official Node.js image.
FROM node:14
# Set the working directory.
WORKDIR /usr/src/app
# Copy package.json and install dependencies.
COPY package*.json ./
RUN npm install
# Copy the rest of the application code.
COPY . .
# Expose the port your app runs on.
EXPOSE 8080
# Start the application.
CMD ["node", "app.js"]
-
Backend microservice:
# Use the official Node.js image.
FROM node:20
# Set the working directory.
WORKDIR /usr/src/app
# Copy package.json and install dependencies.
COPY package*.json ./
RUN npm install
# Copy the rest of the application code.
COPY . .
# Expose the port your app runs on.
EXPOSE 3000
# Start the application.
CMD ["node", "app.js"]
Build Docker images for each service like:
docker build -t my-microservice:latest
Push Docker images to a container registry like Docker Hub or Google Container Registry (GCR):
docker push myusername/my-microservice:latest
Step 2: Deploy Microservices on Kubernetes
-
Create Kubernetes Deployment and Service files for each microservice. Here’s an example for a deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-microservice
spec:
replicas: 2
selector:
matchLabels:
app: my-microservice
template:
metadata:
labels:
app: my-microservice
spec:
containers:
- name: my-microservice
image: myusername/my-microservice:latest
ports:
- containerPort: 8080
-
And a service file for each microservice:
apiVersion: v1
kind: Service
metadata:
name: my-microservice
spec:
type: ClusterIP
selector:
app: my-microservice
ports:
- port: 8080
targetPort: 8080
-
Apply these configurations to your Kubernetes cluster:
kubectl apply -f my-microservice-deployment.yaml
kubectl apply -f my-microservice-service.yaml
Step 3: Enable Auto-Scaling
-
Set up the Horizontal Pod Autoscaler (HPA) to automatically scale the number of pods in your deployment based on CPU usage:
kubectl autoscale deployment my-microservice --cpu-percent=50 --min=1 --max=10
Monitor the HPA using:
kubectl get hpa
This will show you the current CPU usage and the desired number of replicas.
Step 4: Now expose the services via NGINX-Ingress
-
Install the nginx-ingress service via helm chart from which we can expose application for the access from browser:
Step 1: Add the NGINX Ingress Helm repository
Run the following command to add the NGINX Ingress Helm repository:
helm repo add nginx-stable https://helm.nginx.com/stable
Step 2: Update the Helm repository
Run the following command to update the Helm repository:
helm repo update
Step 3: Install the NGINX Ingress Controller
Run the following command to install the NGINX Ingress Controller:
helm install nginx-ingress nginx-stable/nginx-ingress
Now create file named “ingress.yaml” to apply in the kubernete:
apiVersion: networking.k8s.io/v1beta1
kind: Ingress
metadata:
name: netflix-ingress
spec:
tls:
- hosts:
- frontend.example.com
secretName: frontend-tls
- hosts:
- backend.example.com
secretName: backed-tls
- hosts:
- admin.example.com
secretName: admin-tls
rules:
- host: frontend.example.com
http:
paths:
- path: /
backend:
serviceName: frontend
servicePort: 8080
- host: frontend.example.com
http:
paths:
- path: /
backend:
serviceName: backend
servicePort: 3000
- host: backend.example.com
http:
paths:
- path: /
backend:
serviceName: admin
servicePort: 5000
Install the SSL certificates:
You'll need to create a Kubernetes secret for each SSL certificate, using the following command:
kubectl create secret tls frontend-tls --key /certs/frontend.key --cert /certs/frontend.crt
kubectl create secret tls backend-tls --key /certs/backend.key --cert /certs/backend.crt
kubectl create secret tls admin-tls --key /certs/admin.key --cert /certs/admin.crt
Finally apply the ingress.yaml
kubectl apply -f ingress.yaml
Step 4: Test and Monitor
- Test your deployment by simulating load using tools like Apache JMeter or Locust to ensure that the auto-scaling works as expected.
- Monitor your cluster with the Kubernetes dashboard or other monitoring tools like Prometheus and Grafana to get insights into the performance and health of your services.
Conclusion
By now, you’ve seen how Kubernetes can transform the way you deploy and manage your microservices. From containerizing your applications to setting up auto-scaling and traffic management, Kubernetes offers the tools you need to handle modern workloads with ease.
Implementing these practices will not only enhance your application's performance but also streamline your deployment processes, making it easier to scale and adapt to changing demands.
Ready to leverage Kubernetes for your next project? Dive into the details, experiment with the configurations, and watch your applications thrive. For more tips, resources, and expert guidance, stay tuned to our blog or contact us for personalized support. Happy deploying!
If you need professional assistance with cloud and DevOps solutions, explore our cloud and DevOps services to see how we can help you streamline your operations and maximize performance.
Share to:
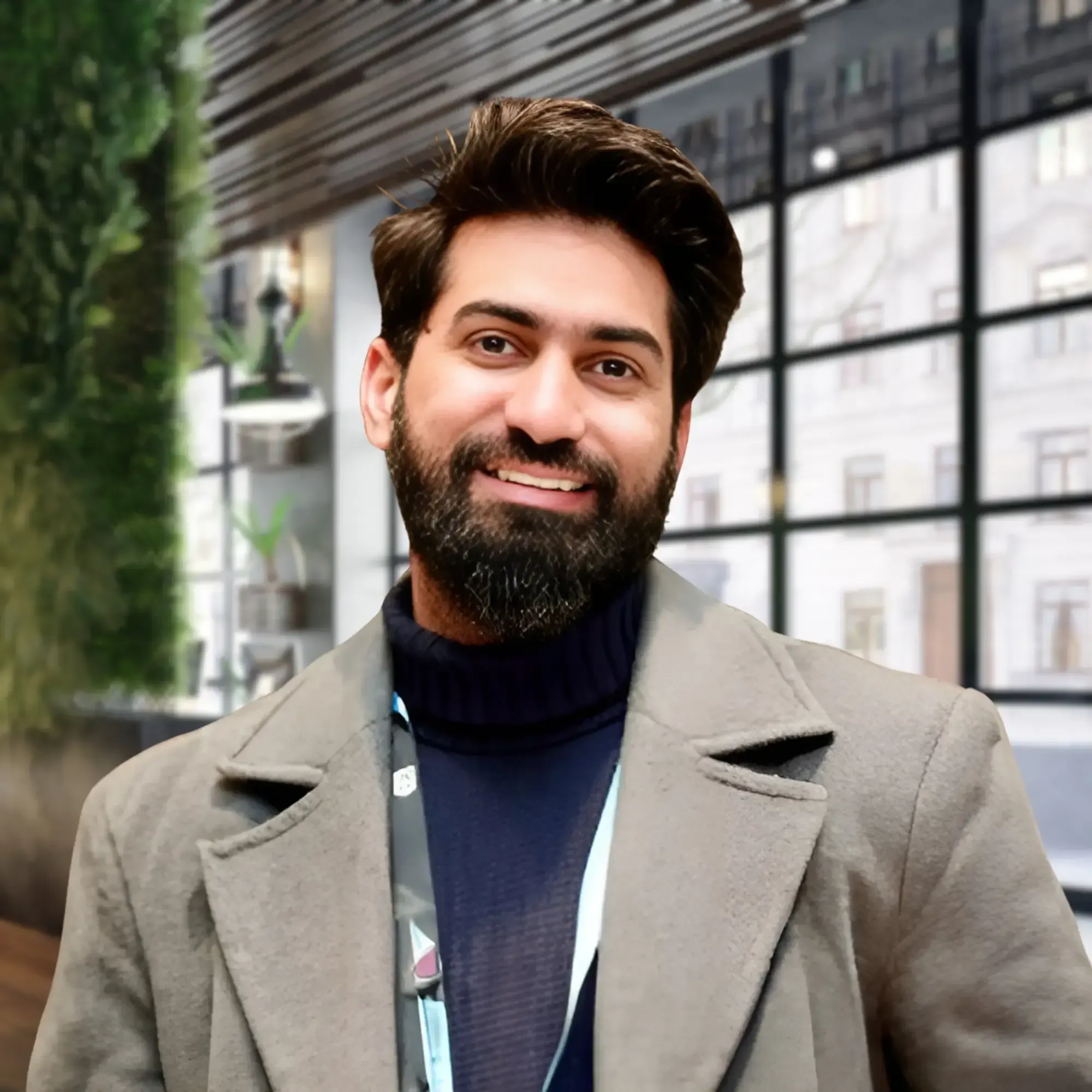
Written By:
Furqan AzizFurqan Aziz is CEO & Founder of InvoZone. He is a tech enthusiast by heart with 10+ years ... Know more
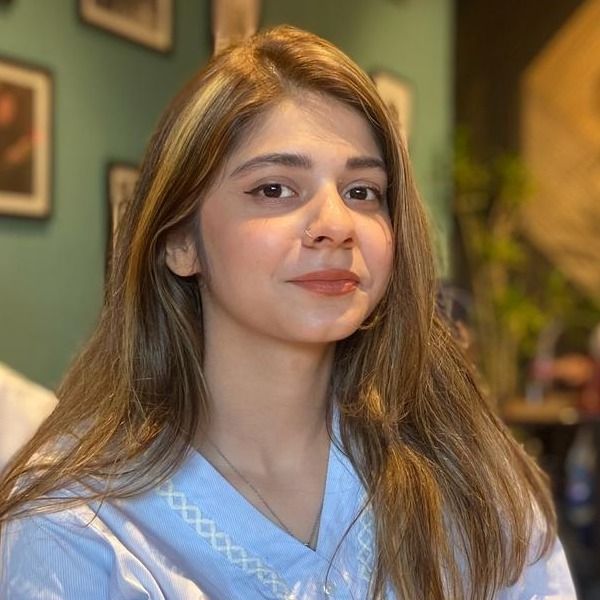
Contributed By:
Senior Content Lead
Get Help From Experts At InvoZone In This Domain