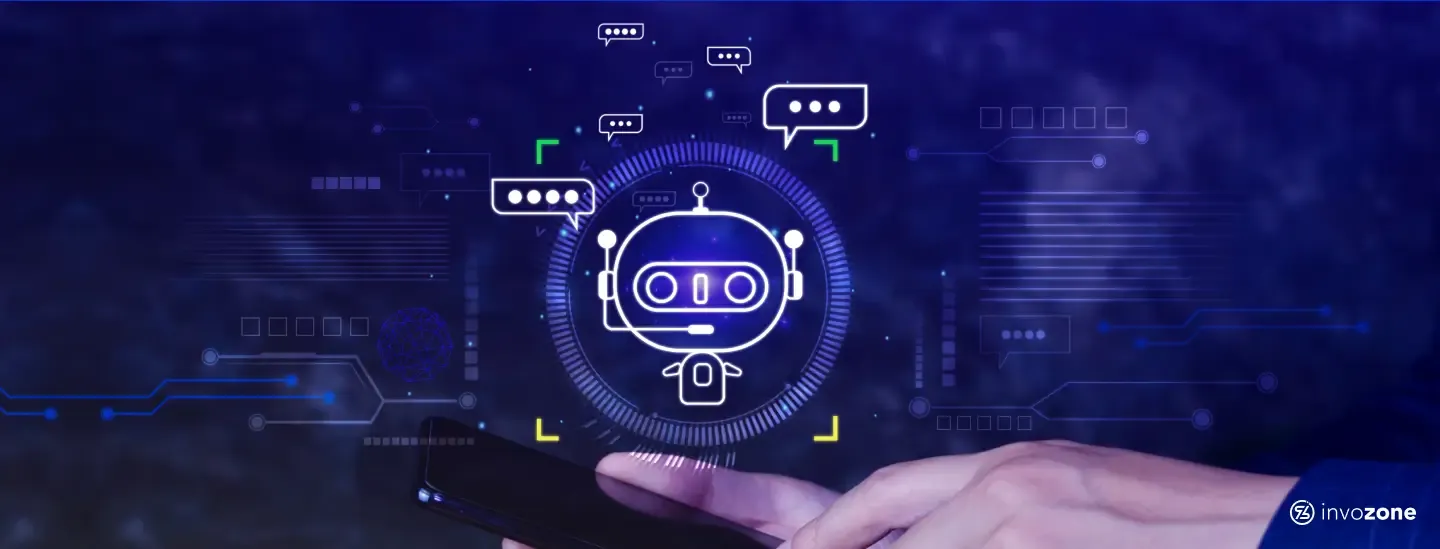
How to use OpenAI API to Build a Sentiment Analyzer in a Node.js App
This blog teaches you how to build a sentiment analyzer with OpenAI’s API and Node.js. You’ll set up a Node.js project, integrate the OpenAI API, and create an application that can categorize text into Positive, Negative, or Neutral sentiments. Perfect for turning text into actionable insights!
Published On: 03 September, 2024
3 min read
Table of Contents
In our tech-driven world, understanding what people think and feel through their words can give us a significant edge, whether we're managing a business or just curious about public opinion. Sentiment analysis is a powerful tool that lets us break down and interpret these sentiments, turning plain text into valuable insights.
In this guide, we’ll walk you through how to build a sentiment analyzer using OpenAI’s API and Node.js. By the end of this tutorial, you’ll have a simple yet effective tool that can classify text as 'Positive', 'Negative', or 'Neutral,' helping you make sense of the messages you encounter.
Prerequisites
Before starting, ensure you have the following:
- OpenAI API Key: Sign up at OpenAI and obtain an API key.
- Node.js Installed: Ensure Node.js is installed on your machine.
- Basic Knowledge of JavaScript and Node.js: Familiarity with these technologies will be beneficial.
Step 1: Setting Up the Node.js Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir sentiment-analyzer
cd sentiment-analyzer
npm init -y
This initializes a new Node.js project with a package.json file.
Step 2: Install Required Packages
You need to install the axios package to make HTTP requests and dotenv to manage environment variables:
npm install axios dotenv
Step 3: Set Up Environment Variables
Create a .env file in the root of your project to store your OpenAI API key:
OPENAI_API_KEY=your-openai-api-key
Make sure to replace your-openai-api-key with the actual API key you obtained from OpenAI.
Step 4: Writing the Code for Sentiment Analysis
Create a new file index.js to handle the logic for sentiment analysis.
- Load Environment Variables and Import Required Modules:
require('dotenv').config();
const axios = require('axios');
This code loads the environment variables from the .env file and imports the axios library for making HTTP requests.
-
Set Up the OpenAI API Request:
Create a function that sends a request to the OpenAI API to analyze sentiment:
async function analyzeSentiment(text) {
const apiKey = process.env.OPENAI_API_KEY;
try {
const response = await axios.post(
'https://api.openai.com/v1/completions',
{
model: 'text-davinci-003',
prompt: `Analyze the sentiment of the following text: "${text}". Respond with 'Positive', 'Negative', or 'Neutral'.`,
max_tokens: 10,
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json',
},
}
);
const sentiment = response.data.choices[0].text.trim();
return sentiment;
} catch (error) {
console.error('Error analyzing sentiment:', error.response.data);
return 'Error';
}
}
This function does the following:
- API Key: It retrieves the API key from the environment variables.
- API Request: It makes a POST request to the OpenAI API, using the text-davinci-003 model to analyze the sentiment of the input text.
- Prompt: The prompt asks the model to classify the sentiment as 'Positive', 'Negative', or 'Neutral'.
- Response Handling: It returns the sentiment classification or an error message if something goes wrong.
- Create an Endpoint to Handle Sentiment Analysis Requests:
Set up an Express server to handle incoming requests for sentiment analysis:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/analyze-sentiment', async (req, res) => {
const { text } = req.body;
if (!text) {
return res.status(400).send({ error: 'Text is required' });
}
const sentiment = await analyzeSentiment(text);
res.send({ sentiment });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code sets up an Express server with a POST endpoint at /analyze-sentiment. The server expects a JSON body with a text field, which it sends to the analyzeSentiment function. The result is then returned to the client.
Step 5: Running and Testing the Application
To start your server and test the sentiment analysis feature:
- Start the Server:
node index.js
- Test the API:
Use a tool like Postman or curl to test the API:
curl -X POST http://localhost:3000/analyze-sentiment -H "Content-Type: application/json" -d '{"text": "I love coding in Node.js!"}'
If everything is set up correctly, you should receive a response indicating whether the sentiment of the text is 'Positive', 'Negative', or 'Neutral'.
Step 6: Areas to Be Careful About
- API Costs: OpenAI charges for API usage, so be mindful of the costs associated with running sentiment analysis, especially for large-scale applications.
- Rate Limits: OpenAI enforces rate limits on their API, so ensure your application handles potential rate limit errors gracefully.
- Text Input Validation: Validate the text input to avoid sending unnecessary requests to the API.
Build Your Sentiment Analyzer Today!
Our Easy Guide Shows You How To Create A Sentiment Analyzer Using OpenAI's API and Node.js.
Get Started NowConclusion
And there you have it! You've successfully set up a sentiment analyzer that uses OpenAI’s technology to understand and categorize text sentiment. This tool is a great start for analyzing feedback, reviews, or social media posts, giving you a clearer picture of what people are really saying.
Remember to keep an eye on your API usage and handle any potential issues like rate limits and input validation. With this setup, you’re now equipped to dive deeper into text analysis and uncover more from your data. Happy analyzing!
Don’t Have Time To Read Now? Download It For Later.
Table of Contents
In our tech-driven world, understanding what people think and feel through their words can give us a significant edge, whether we're managing a business or just curious about public opinion. Sentiment analysis is a powerful tool that lets us break down and interpret these sentiments, turning plain text into valuable insights.
In this guide, we’ll walk you through how to build a sentiment analyzer using OpenAI’s API and Node.js. By the end of this tutorial, you’ll have a simple yet effective tool that can classify text as 'Positive', 'Negative', or 'Neutral,' helping you make sense of the messages you encounter.
Prerequisites
Before starting, ensure you have the following:
- OpenAI API Key: Sign up at OpenAI and obtain an API key.
- Node.js Installed: Ensure Node.js is installed on your machine.
- Basic Knowledge of JavaScript and Node.js: Familiarity with these technologies will be beneficial.
Step 1: Setting Up the Node.js Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir sentiment-analyzer
cd sentiment-analyzer
npm init -y
This initializes a new Node.js project with a package.json file.
Step 2: Install Required Packages
You need to install the axios package to make HTTP requests and dotenv to manage environment variables:
npm install axios dotenv
Step 3: Set Up Environment Variables
Create a .env file in the root of your project to store your OpenAI API key:
OPENAI_API_KEY=your-openai-api-key
Make sure to replace your-openai-api-key with the actual API key you obtained from OpenAI.
Step 4: Writing the Code for Sentiment Analysis
Create a new file index.js to handle the logic for sentiment analysis.
- Load Environment Variables and Import Required Modules:
require('dotenv').config();
const axios = require('axios');
This code loads the environment variables from the .env file and imports the axios library for making HTTP requests.
-
Set Up the OpenAI API Request:
Create a function that sends a request to the OpenAI API to analyze sentiment:
async function analyzeSentiment(text) {
const apiKey = process.env.OPENAI_API_KEY;
try {
const response = await axios.post(
'https://api.openai.com/v1/completions',
{
model: 'text-davinci-003',
prompt: `Analyze the sentiment of the following text: "${text}". Respond with 'Positive', 'Negative', or 'Neutral'.`,
max_tokens: 10,
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json',
},
}
);
const sentiment = response.data.choices[0].text.trim();
return sentiment;
} catch (error) {
console.error('Error analyzing sentiment:', error.response.data);
return 'Error';
}
}
This function does the following:
- API Key: It retrieves the API key from the environment variables.
- API Request: It makes a POST request to the OpenAI API, using the text-davinci-003 model to analyze the sentiment of the input text.
- Prompt: The prompt asks the model to classify the sentiment as 'Positive', 'Negative', or 'Neutral'.
- Response Handling: It returns the sentiment classification or an error message if something goes wrong.
- Create an Endpoint to Handle Sentiment Analysis Requests:
Set up an Express server to handle incoming requests for sentiment analysis:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/analyze-sentiment', async (req, res) => {
const { text } = req.body;
if (!text) {
return res.status(400).send({ error: 'Text is required' });
}
const sentiment = await analyzeSentiment(text);
res.send({ sentiment });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code sets up an Express server with a POST endpoint at /analyze-sentiment. The server expects a JSON body with a text field, which it sends to the analyzeSentiment function. The result is then returned to the client.
Step 5: Running and Testing the Application
To start your server and test the sentiment analysis feature:
- Start the Server:
node index.js
- Test the API:
Use a tool like Postman or curl to test the API:
curl -X POST http://localhost:3000/analyze-sentiment -H "Content-Type: application/json" -d '{"text": "I love coding in Node.js!"}'
If everything is set up correctly, you should receive a response indicating whether the sentiment of the text is 'Positive', 'Negative', or 'Neutral'.
Step 6: Areas to Be Careful About
- API Costs: OpenAI charges for API usage, so be mindful of the costs associated with running sentiment analysis, especially for large-scale applications.
- Rate Limits: OpenAI enforces rate limits on their API, so ensure your application handles potential rate limit errors gracefully.
- Text Input Validation: Validate the text input to avoid sending unnecessary requests to the API.
Build Your Sentiment Analyzer Today!
Our Easy Guide Shows You How To Create A Sentiment Analyzer Using OpenAI's API and Node.js.
Get Started NowConclusion
And there you have it! You've successfully set up a sentiment analyzer that uses OpenAI’s technology to understand and categorize text sentiment. This tool is a great start for analyzing feedback, reviews, or social media posts, giving you a clearer picture of what people are really saying.
Remember to keep an eye on your API usage and handle any potential issues like rate limits and input validation. With this setup, you’re now equipped to dive deeper into text analysis and uncover more from your data. Happy analyzing!
Share to:
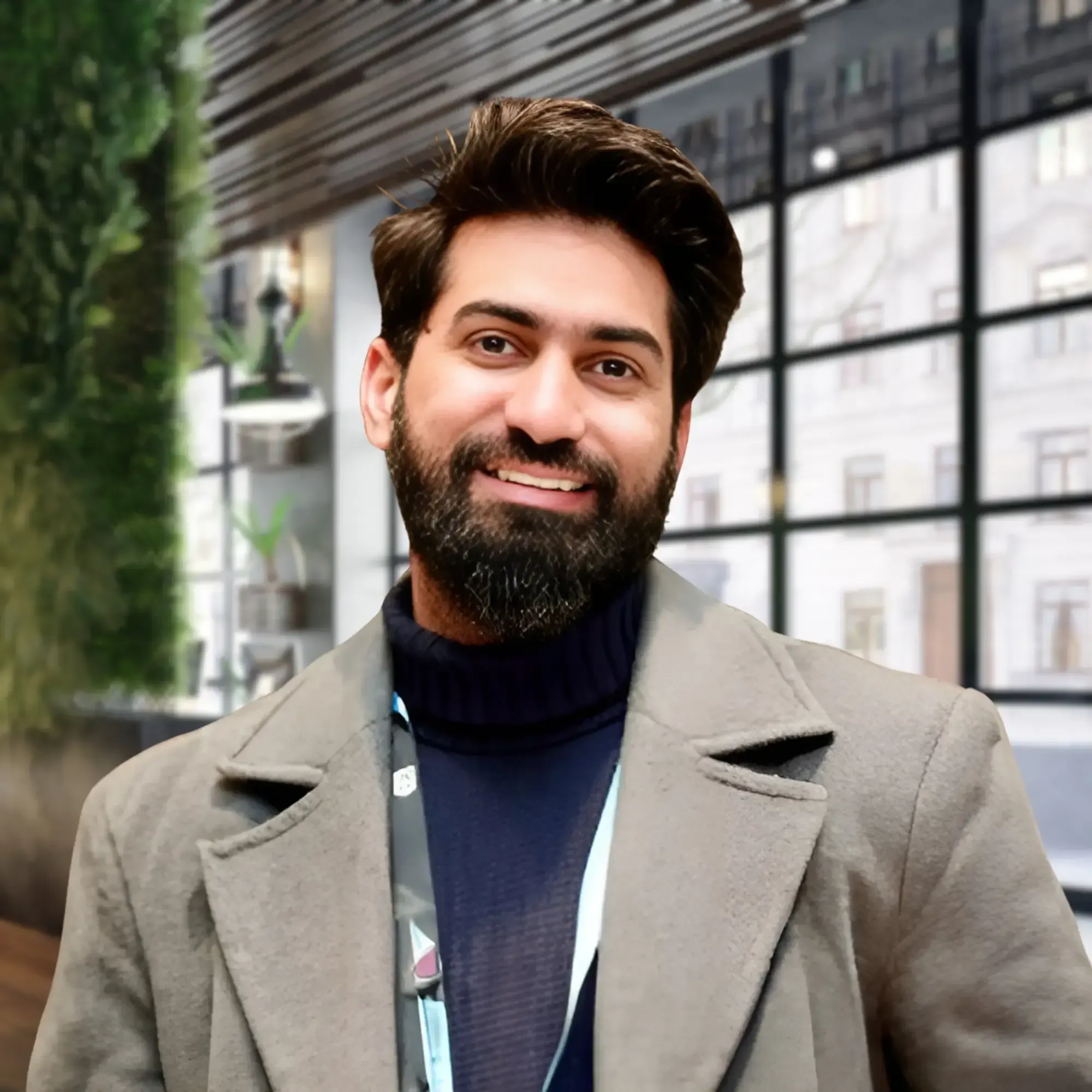
Written By:
Furqan AzizFurqan Aziz is CEO & Founder of InvoZone. He is a tech enthusiast by heart with 10+ years ... Know more
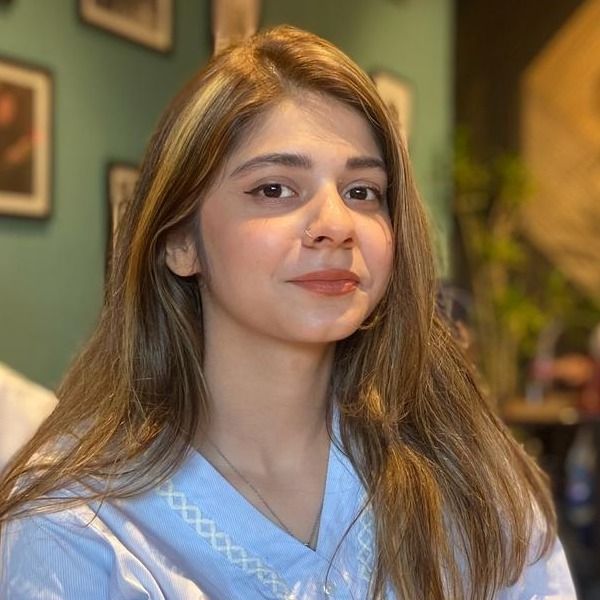
Contributed By:
Senior Content Lead
Get Help From Experts At InvoZone In This Domain