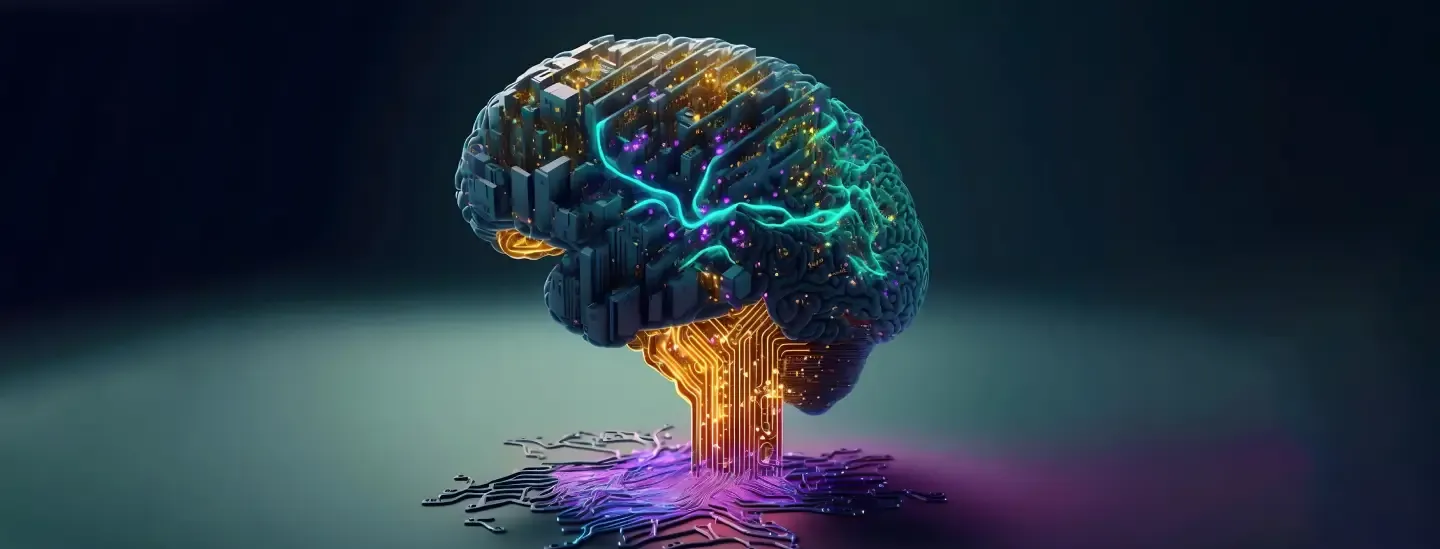
Training LLMs Models and The Cost of Fine-Tuning LLMs
This blog discusses the importance of fine-tuning large language models (LLMs) for specific tasks, the costs involved, and various techniques such as supervised fine-tuning and few-shot learning. It provides a step-by-step guide to fine-tuning, along with best practices for effective implementation.
Published On: 04 March, 2025
4 min read
Table of Contents
I read about a company that was building a chatbot for a niche customer service application. And while they were in development process, pretty soon they realized that their pre-trained language model, which worked fine for general tasks, was struggling hard when it came to understanding the specifics of their industry’s terminology. The solution? Fine-tuning.
This process involved taking the model and training it further on a specialized dataset, one full of examples from the industry in question, so it could handle the unique challenges of the job.
If you're looking into how large language models like GPT operate, you'll find that they rely on extensive text data for training, which helps them understand language and generate coherent responses. However, fine-tuning these models for specific applications can be quite expensive. It requires significant computational power and resources, which can strain budgets for many organizations. While improving a model's performance is valuable, the costs involved in fine-tuning often prompt discussions about accessibility in the field of AI development.
Our Experts Help You Optimize Models Efficiently While Reducing Costs
Train Smarter With Our ExpertsWhat Are LLMs and How Do They Work?
At their core, LLMs are powerful algorithms designed to understand and generate human-like text. They're built on a structure known as the Transformer architecture, which allows them to predict the next word in a sentence based on the context they’ve already seen. These models get smarter by being trained on vast amounts of text data, which lets them understand language rules and nuances.
Models like GPT (Generative Pre-trained Transformers) are great examples. They start by being pre-trained on large, general datasets, learning patterns, sentence structures, and the way words are used in context. The result? A model that can create text, answer questions, and even translate languages. But here’s the catch: while they’re excellent at general tasks, they may not always perform well on specific jobs.
What Is Fine-Tuning It and Why Do We Need It?
Fine-tuning is the process of taking an already trained model (like GPT) and tweaking it to perform a specific task better. For example, while a pre-trained model can generate text, you might need it to specialize in understanding sentiment in customer reviews or picking up trends in tweets. That's where fine-tuning comes in.
Why should you fine-tune? Simple. Pre-trained models are usually trained on generic data. So, they do a great job at handling broad tasks but might struggle with domain-specific tasks, like detecting sarcasm in customer feedback or summarizing technical research papers. Fine-tuning helps you make the model more accurate for your specific needs without starting from scratch.
Different Ways to Fine-Tune LLMs
Fine-tuning isn’t a one-size-fits-all approach. Depending on your goals, there are different ways to fine-tune your model:
- Supervised Fine-Tuning
This is the most common method. Here, you train the model using a dataset that’s already labeled with the right answers. For example, if you're working on a sentiment analysis task, you’ll use a dataset where each text is labeled as "positive," "negative," or "neutral."
- Few-shot Learning
Sometimes, gathering tons of labeled data isn’t practical. Few-shot learning helps by providing just a few examples of the task. This is useful when you don’t have a large dataset to work with but still want to guide the model to the right output.
- Transfer Learning
Transfer learning allows the model to use what it has learned from one task and apply that knowledge to a new, but related task. For instance, you might fine-tune a general language model to perform better at legal document analysis.
- Domain-Specific Fine-Tuning
This is when you take a pre-trained model and fine-tune it to a specific field. Let’s say you’re building a chatbot for a hospital. By fine-tuning your model with medical records, it becomes more capable of understanding medical jargon and providing accurate responses within that domain.
How to Fine-Tune LLMs Step-by-Step
Now let’s get to the fun part: how to fine-tune an LLM. To make it simple, let’s use an example. Suppose we’re working with a pre-trained GPT-2 model, and we want it to perform sentiment analysis on tweets. Here’s how you’d go about fine-tuning it:
Step 1: Choose a Model and Dataset
The first thing you need is a pre-trained model. In this case, we'll use GPT-2, available through Hugging Face. Then, you’ll need a dataset for the task at hand. For sentiment analysis, you could use a dataset of tweets labeled by sentiment (positive, neutral, negative).
Step 2: Load Your Dataset
Now, you need to import your dataset. You can use libraries like Hugging Face's datasets library to load publicly available datasets. For instance, you could use a dataset like Tweet Sentiment Extraction.
from datasets import load_dataset
dataset = load_dataset("mteb/tweet_sentiment_extraction")
Once you load the dataset, you’ll get your training and testing data in a format that’s easy to use.
Step 3: Tokenize Your Data
Since LLMs work with tokens (small chunks of text), you need to tokenize your data. You’ll use a tokenizer that matches your model (e.g., GPT-2 tokenizer).
from transformers import GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained("gpt2")
This tokenizer will break down your sentences into tokens that the model can understand. After tokenizing the dataset, you can create training and test sets.
Step 4: Set Up the Model
Now, load the model you’re going to fine-tune. For sentiment analysis, you would load GPT-2 with a classification head.
from transformers import GPT2ForSequenceClassification
model = GPT2ForSequenceClassification.from_pretrained("gpt2", num_labels=3)
This step prepares the model to handle your task of classifying text into three categories: positive, negative, or neutral.
Step 5: Train the Model
Now it’s time to fine-tune the model. You’ll use the Hugging Face Trainer class to handle this. You’ll need to set up a few things, like training arguments (batch size, number of epochs, etc.).
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir="./results",
num_train_epochs=3,
per_device_train_batch_size=16,
per_device_eval_batch_size=64,
)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=dataset["train"],
eval_dataset=dataset["test"],
)
trainer.train()
That’s it! The model is now fine-tuned and ready to perform sentiment analysis.
Step 6: Evaluate the Model
After fine-tuning, it’s essential to evaluate how well the model is performing. You can do this using a validation or test set, and check metrics like accuracy to see how well it classifies sentiment.
trainer.evaluate()
The Cost of Fine-Tuning LLMs
Fine-tuning sounds exciting, but let’s talk about the elephant in the room: cost. Training a model, especially large ones like GPT-2, is computationally expensive. Fine-tuning involves running a model through thousands of iterations to learn from your data, and this requires substantial computing power.
You’ll likely need access to high-performance GPUs or specialized hardware like TPUs (Tensor Processing Units). If you’re working with a cloud service like AWS, Google Cloud, or Azure, the costs can quickly add up, depending on how long you train the model and the resources required.
So, how much does it cost? It depends. The price can vary based on:
- The size of your dataset
- The model you're using
- The time it takes to fine-tune
But expect to pay a premium if you need to use powerful machines to fine-tune a large model for an extended period. Fine-tuning can cost anywhere from a few dollars to thousands of dollars if you're training for a long time or using a lot of resources.
Best Practices for Fine-Tuning LLMs
To get the best results and avoid blowing your budget, here are a few tips:
- Start Small: Begin with a smaller dataset and fewer epochs to test how the model is performing. You can always scale up later.
- Monitor Training: Keep an eye on training performance to make sure you’re not overfitting or underfitting.
- Use Pre-built Datasets: When possible, use publicly available datasets to avoid the time and cost of creating one from scratch.
- Hyperparameter Tuning: Experiment with different learning rates, batch sizes, and other hyperparameters to find the best setup for your task.
Conclusion
Fine-tuning LLMs takes effort, resources, and a clear plan. A general model can handle many tasks, but if you need something that understands your industry or specific data better, fine-tuning is the way to go.
The key is to approach it wisely. Start small, test results, and adjust as needed. Some projects may need just a little fine-tuning, while others require more training time and computing power. Either way, the goal is simple “making the model more useful for your needs”
AI keeps advancing, and every step forward brings new possibilities. Fine-tuning isn’t always necessary, but for those who need more accuracy and relevance, it’s an option worth considering.
Don’t Have Time To Read Now? Download It For Later.
Table of Contents
I read about a company that was building a chatbot for a niche customer service application. And while they were in development process, pretty soon they realized that their pre-trained language model, which worked fine for general tasks, was struggling hard when it came to understanding the specifics of their industry’s terminology. The solution? Fine-tuning.
This process involved taking the model and training it further on a specialized dataset, one full of examples from the industry in question, so it could handle the unique challenges of the job.
If you're looking into how large language models like GPT operate, you'll find that they rely on extensive text data for training, which helps them understand language and generate coherent responses. However, fine-tuning these models for specific applications can be quite expensive. It requires significant computational power and resources, which can strain budgets for many organizations. While improving a model's performance is valuable, the costs involved in fine-tuning often prompt discussions about accessibility in the field of AI development.
Our Experts Help You Optimize Models Efficiently While Reducing Costs
Train Smarter With Our ExpertsWhat Are LLMs and How Do They Work?
At their core, LLMs are powerful algorithms designed to understand and generate human-like text. They're built on a structure known as the Transformer architecture, which allows them to predict the next word in a sentence based on the context they’ve already seen. These models get smarter by being trained on vast amounts of text data, which lets them understand language rules and nuances.
Models like GPT (Generative Pre-trained Transformers) are great examples. They start by being pre-trained on large, general datasets, learning patterns, sentence structures, and the way words are used in context. The result? A model that can create text, answer questions, and even translate languages. But here’s the catch: while they’re excellent at general tasks, they may not always perform well on specific jobs.
What Is Fine-Tuning It and Why Do We Need It?
Fine-tuning is the process of taking an already trained model (like GPT) and tweaking it to perform a specific task better. For example, while a pre-trained model can generate text, you might need it to specialize in understanding sentiment in customer reviews or picking up trends in tweets. That's where fine-tuning comes in.
Why should you fine-tune? Simple. Pre-trained models are usually trained on generic data. So, they do a great job at handling broad tasks but might struggle with domain-specific tasks, like detecting sarcasm in customer feedback or summarizing technical research papers. Fine-tuning helps you make the model more accurate for your specific needs without starting from scratch.
Different Ways to Fine-Tune LLMs
Fine-tuning isn’t a one-size-fits-all approach. Depending on your goals, there are different ways to fine-tune your model:
- Supervised Fine-Tuning
This is the most common method. Here, you train the model using a dataset that’s already labeled with the right answers. For example, if you're working on a sentiment analysis task, you’ll use a dataset where each text is labeled as "positive," "negative," or "neutral."
- Few-shot Learning
Sometimes, gathering tons of labeled data isn’t practical. Few-shot learning helps by providing just a few examples of the task. This is useful when you don’t have a large dataset to work with but still want to guide the model to the right output.
- Transfer Learning
Transfer learning allows the model to use what it has learned from one task and apply that knowledge to a new, but related task. For instance, you might fine-tune a general language model to perform better at legal document analysis.
- Domain-Specific Fine-Tuning
This is when you take a pre-trained model and fine-tune it to a specific field. Let’s say you’re building a chatbot for a hospital. By fine-tuning your model with medical records, it becomes more capable of understanding medical jargon and providing accurate responses within that domain.
How to Fine-Tune LLMs Step-by-Step
Now let’s get to the fun part: how to fine-tune an LLM. To make it simple, let’s use an example. Suppose we’re working with a pre-trained GPT-2 model, and we want it to perform sentiment analysis on tweets. Here’s how you’d go about fine-tuning it:
Step 1: Choose a Model and Dataset
The first thing you need is a pre-trained model. In this case, we'll use GPT-2, available through Hugging Face. Then, you’ll need a dataset for the task at hand. For sentiment analysis, you could use a dataset of tweets labeled by sentiment (positive, neutral, negative).
Step 2: Load Your Dataset
Now, you need to import your dataset. You can use libraries like Hugging Face's datasets library to load publicly available datasets. For instance, you could use a dataset like Tweet Sentiment Extraction.
from datasets import load_dataset
dataset = load_dataset("mteb/tweet_sentiment_extraction")
Once you load the dataset, you’ll get your training and testing data in a format that’s easy to use.
Step 3: Tokenize Your Data
Since LLMs work with tokens (small chunks of text), you need to tokenize your data. You’ll use a tokenizer that matches your model (e.g., GPT-2 tokenizer).
from transformers import GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained("gpt2")
This tokenizer will break down your sentences into tokens that the model can understand. After tokenizing the dataset, you can create training and test sets.
Step 4: Set Up the Model
Now, load the model you’re going to fine-tune. For sentiment analysis, you would load GPT-2 with a classification head.
from transformers import GPT2ForSequenceClassification
model = GPT2ForSequenceClassification.from_pretrained("gpt2", num_labels=3)
This step prepares the model to handle your task of classifying text into three categories: positive, negative, or neutral.
Step 5: Train the Model
Now it’s time to fine-tune the model. You’ll use the Hugging Face Trainer class to handle this. You’ll need to set up a few things, like training arguments (batch size, number of epochs, etc.).
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir="./results",
num_train_epochs=3,
per_device_train_batch_size=16,
per_device_eval_batch_size=64,
)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=dataset["train"],
eval_dataset=dataset["test"],
)
trainer.train()
That’s it! The model is now fine-tuned and ready to perform sentiment analysis.
Step 6: Evaluate the Model
After fine-tuning, it’s essential to evaluate how well the model is performing. You can do this using a validation or test set, and check metrics like accuracy to see how well it classifies sentiment.
trainer.evaluate()
The Cost of Fine-Tuning LLMs
Fine-tuning sounds exciting, but let’s talk about the elephant in the room: cost. Training a model, especially large ones like GPT-2, is computationally expensive. Fine-tuning involves running a model through thousands of iterations to learn from your data, and this requires substantial computing power.
You’ll likely need access to high-performance GPUs or specialized hardware like TPUs (Tensor Processing Units). If you’re working with a cloud service like AWS, Google Cloud, or Azure, the costs can quickly add up, depending on how long you train the model and the resources required.
So, how much does it cost? It depends. The price can vary based on:
- The size of your dataset
- The model you're using
- The time it takes to fine-tune
But expect to pay a premium if you need to use powerful machines to fine-tune a large model for an extended period. Fine-tuning can cost anywhere from a few dollars to thousands of dollars if you're training for a long time or using a lot of resources.
Best Practices for Fine-Tuning LLMs
To get the best results and avoid blowing your budget, here are a few tips:
- Start Small: Begin with a smaller dataset and fewer epochs to test how the model is performing. You can always scale up later.
- Monitor Training: Keep an eye on training performance to make sure you’re not overfitting or underfitting.
- Use Pre-built Datasets: When possible, use publicly available datasets to avoid the time and cost of creating one from scratch.
- Hyperparameter Tuning: Experiment with different learning rates, batch sizes, and other hyperparameters to find the best setup for your task.
Conclusion
Fine-tuning LLMs takes effort, resources, and a clear plan. A general model can handle many tasks, but if you need something that understands your industry or specific data better, fine-tuning is the way to go.
The key is to approach it wisely. Start small, test results, and adjust as needed. Some projects may need just a little fine-tuning, while others require more training time and computing power. Either way, the goal is simple “making the model more useful for your needs”
AI keeps advancing, and every step forward brings new possibilities. Fine-tuning isn’t always necessary, but for those who need more accuracy and relevance, it’s an option worth considering.
Frequently Asked Questions
How much does it cost to fine-tune an LLM?
The cost depends on factors like dataset size, model complexity, and training duration. It can range from a few dollars to thousands if using cloud-based GPUs or TPUs.
Is fine-tuning necessary for every LLM application?
Not always. Many tasks can be handled with pre-trained models using prompt engineering or few-shot learning. Fine-tuning is useful for domain-specific applications.
What are the best tools for fine-tuning LLMs?
Popular tools include Hugging Face Transformers, TensorFlow, PyTorch, and cloud services like AWS SageMaker, Google Cloud AI, and Azure ML.
How long does it take to fine-tune an LLM?
Training time varies based on model size and computing power. Small-scale fine-tuning can take hours, while larger models may require days or even weeks.
Share to:
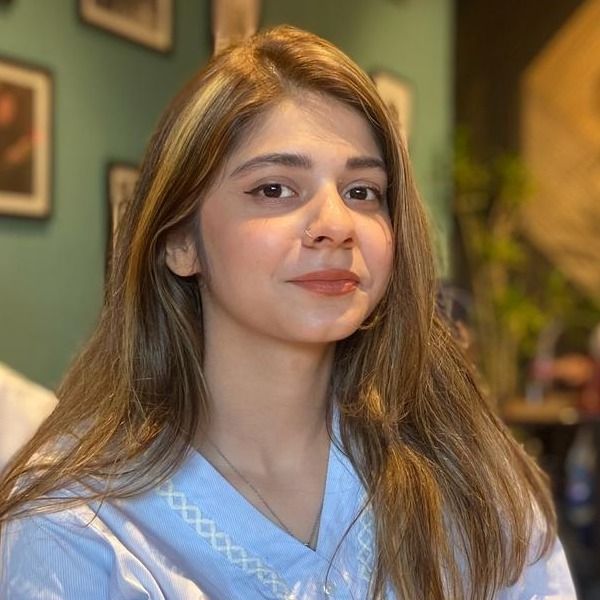
Written By:
Harram ShahidHarram is like a walking encyclopedia who loves to write about various genres but at the t... Know more
Get Help From Experts At InvoZone In This Domain