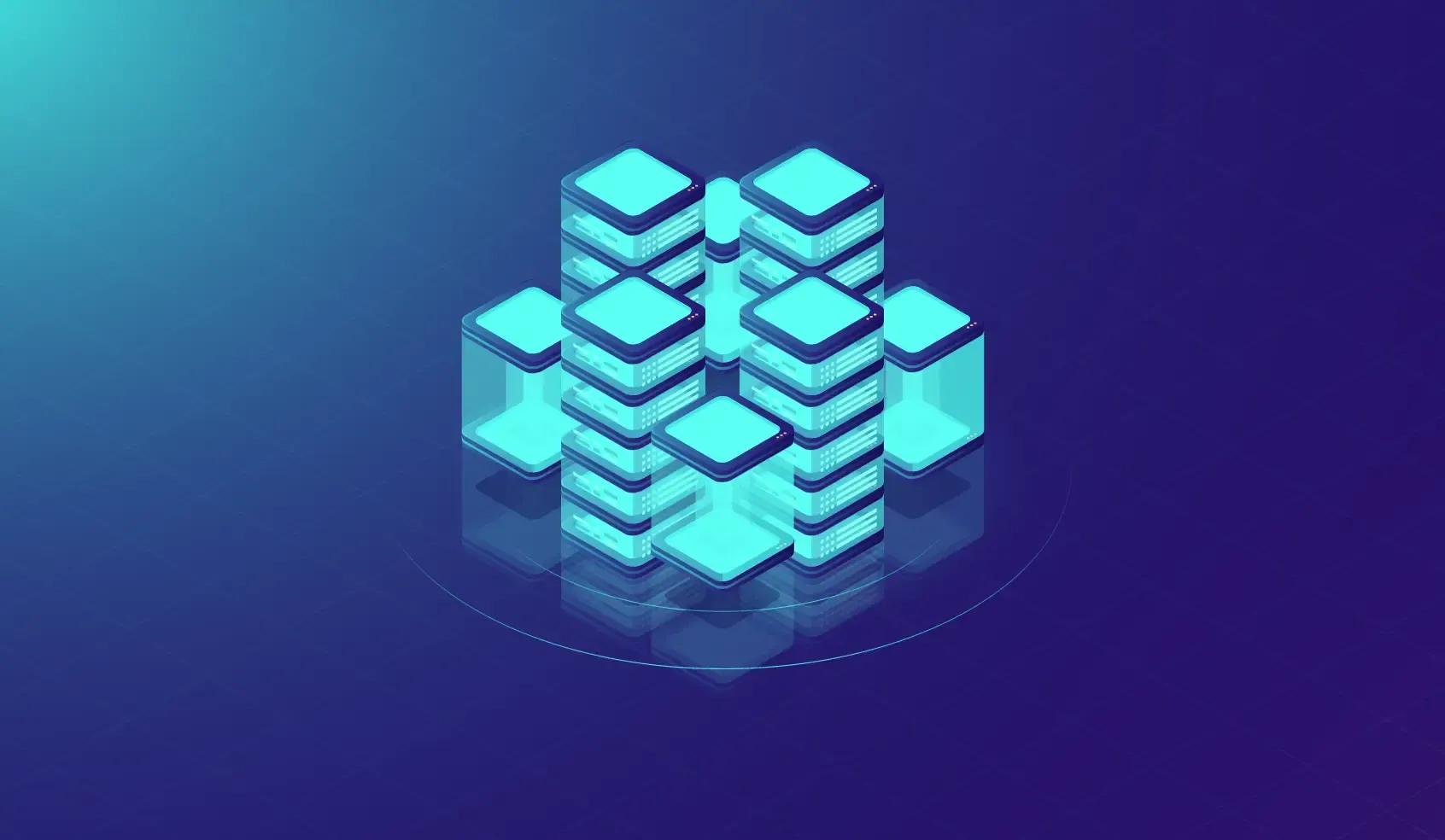
Building Scalable Microservices Architecture: A Step-by-Step Guide
This blog provides a comprehensive, step-by-step guide to creating a scalable microservices architecture. It covers everything from designing services to deploying with Docker, ensuring you understand how to build resilient applications that can grow with your business.
Published On: 30 October, 2024
3 min read
Table of Contents
If you've ever found yourself frustrated with the limitations of traditional monolithic applications, where everything is squished together and scaling feels like a Herculean task, you’re not alone. Those days of “let’s just throw everything into one big box” are fading fast. Instead, we’re adopting a more flexible and manageable approach: microservices architecture.
Picture this: instead of one giant application that’s tough to modify, you have a collection of smaller services that can be developed, deployed, and scaled independently. This structure allows teams to work concurrently, innovate, and fix issues without bringing the entire system to a halt.
In this guide, we’re going to roll up our sleeves and build a basic microservices application together. No matter if you’re just starting your coding career or you’ve been around the block a few times, I promise there’s something here for you. By the time we’re done, you’ll not only have a working application, but you’ll also walk away with a clearer understanding of how to make your systems scalable and resilient. So, grab your favorite drink, get comfy, and let’s dive into this exciting adventure!
What are Microservices?
Microservices is an architectural style that structures an application as a collection of loosely coupled services. Each service represents a single, business-related function, and can be developed, deployed, and scaled independently of the others. This approach allows teams to work on different parts of the application simultaneously and update components without affecting the entire system.
Key Advantages of Microservices Architecture
Microservices |
Monolithic |
Scalable by design |
Hard to scale |
Loosely coupled components |
Tightly coupled modules |
Easier deployment |
Complex deployment |
Fault isolation |
Single point of failure |
Easier to understand small services |
The entire system needs a deep understanding |
As you can see, the advantages of using microservices far outweigh those of traditional monolithic structures, especially when it comes to scalability and fault tolerance.
Tools and Technologies You’ll Need:
- Node.js for building microservices
- Express.js for routing
- MongoDB for database services
- Docker for containerization
- Kubernetes (optional) for scaling and orchestration
Step-by-Step Tutorial: Building the Microservices
Step 1: Designing the Services
First, let’s plan our microservices architecture. For this example, let’s assume we’re building a simple e-commerce platform. We’ll break it into the following services:
- User Service: Manages user registration and authentication.
- Product Service: Handles product information.
- Order Service: Manages orders and payments.
You can use a simple diagram to visualize the flow of data between these services.
Step 2: Building the API Gateway
An API Gateway acts as the entry point for all client requests. It routes incoming traffic to the correct microservice. We’ll use Express Gateway for this:
npm install -g express-gateway
eg generate my-gateway
cd my-gateway
npm start
This simple setup creates an API Gateway that listens on port 8080. You can now configure it to route requests to the respective microservices.
Step 3: Setting Up the Services with Node.js and Express
Let’s create the User Service first. For this, we’ll need Node.js and Express:
mkdir user-service
cd user-service
npm init -y
npm install express mongoose
Now, create a basic Express server:
const express = require('express');
const mongoose = require('mongoose');
const app = express();
app.use(express.json());
mongoose.connect('mongodb://localhost:27017/users', { useNewUrlParser: true, useUnifiedTopology: true });
const userSchema = new mongoose.Schema({
username: String,
password: String
});
const User = mongoose.model('User', userSchema);
app.post('/register', async (req, res) => {
const user = new User(req.body);
await user.save();
res.send('User registered');
});
app.listen(3001, () => {
console.log('User service running on port 3001');
});
This sets up the user registration service. You can repeat similar steps for Product and Order services.
Step 4: Creating a Centralized Database with MongoDB
Each service can have its own database, but for simplicity, we’ll create one central database using MongoDB to handle all services.
Install MongoDB locally or use a cloud service like MongoDB Atlas. Connect each microservice to this database by replacing the MongoDB URL in each service’s configuration file.
Step 5: Deploying with Docker
We will containerize our services using Docker:
-
Create a
Dockerfile
for each service:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["node", "index.js"]
-
Build and run your Docker containers:
docker build -t user-service .
docker run -p 3001:3001 user-service
Repeat these steps for the other services.
Testing and Scaling the Application
Once your services are running, you can use Postman or cURL to test the endpoints and check if they work together. Scaling is as simple as running more instances of a service. You can do this easily with Docker Compose or Kubernetes.
For example, with Docker Compose, define multiple services in a docker-compose.yml
file and use docker-compose up
to start all services:
version: '3'
services:
user-service:
build: ./user-service
ports:
- "3001:3001"
product-service:
build: ./product-service
ports:
- "3002:3002"
Kubernetes can help you scale further by automating deployment and orchestration, making it easier to handle growing traffic.
Conclusion
Wow, what a journey it’s been! As we finish up this serious plunge into microservices architecture, I hope you’re feeling inspired and equipped to tackle your own projects. Building applications using microservices is about writing code and adopting a mindset that allows you to create systems that can grow and adapt alongside your business needs.
Throughout our time together, we've explored how to design, build, and deploy a microservices application. And while this guide is just a starting point, the world of microservices is vast and full of opportunities. Tools like Kubernetes can take your application to new heights, helping you manage and scale with ease.
If you’re thinking about building a scalable microservices architecture for your business, remember, you don’t have to do it alone. My team and I are here to help you navigate this exciting terrain. Check out our service page to see how we can work together to bring your vision to life. Let’s make something amazing happen!
If you’re looking to build a robust, scalable microservices architecture for your business but need expert guidance, visit my service page to learn how we can help you design, deploy, and maintain enterprise-level microservices systems tailored to your specific needs.
Don’t Have Time To Read Now? Download It For Later.
Table of Contents
If you've ever found yourself frustrated with the limitations of traditional monolithic applications, where everything is squished together and scaling feels like a Herculean task, you’re not alone. Those days of “let’s just throw everything into one big box” are fading fast. Instead, we’re adopting a more flexible and manageable approach: microservices architecture.
Picture this: instead of one giant application that’s tough to modify, you have a collection of smaller services that can be developed, deployed, and scaled independently. This structure allows teams to work concurrently, innovate, and fix issues without bringing the entire system to a halt.
In this guide, we’re going to roll up our sleeves and build a basic microservices application together. No matter if you’re just starting your coding career or you’ve been around the block a few times, I promise there’s something here for you. By the time we’re done, you’ll not only have a working application, but you’ll also walk away with a clearer understanding of how to make your systems scalable and resilient. So, grab your favorite drink, get comfy, and let’s dive into this exciting adventure!
What are Microservices?
Microservices is an architectural style that structures an application as a collection of loosely coupled services. Each service represents a single, business-related function, and can be developed, deployed, and scaled independently of the others. This approach allows teams to work on different parts of the application simultaneously and update components without affecting the entire system.
Key Advantages of Microservices Architecture
Microservices |
Monolithic |
Scalable by design |
Hard to scale |
Loosely coupled components |
Tightly coupled modules |
Easier deployment |
Complex deployment |
Fault isolation |
Single point of failure |
Easier to understand small services |
The entire system needs a deep understanding |
As you can see, the advantages of using microservices far outweigh those of traditional monolithic structures, especially when it comes to scalability and fault tolerance.
Tools and Technologies You’ll Need:
- Node.js for building microservices
- Express.js for routing
- MongoDB for database services
- Docker for containerization
- Kubernetes (optional) for scaling and orchestration
Step-by-Step Tutorial: Building the Microservices
Step 1: Designing the Services
First, let’s plan our microservices architecture. For this example, let’s assume we’re building a simple e-commerce platform. We’ll break it into the following services:
- User Service: Manages user registration and authentication.
- Product Service: Handles product information.
- Order Service: Manages orders and payments.
You can use a simple diagram to visualize the flow of data between these services.
Step 2: Building the API Gateway
An API Gateway acts as the entry point for all client requests. It routes incoming traffic to the correct microservice. We’ll use Express Gateway for this:
npm install -g express-gateway
eg generate my-gateway
cd my-gateway
npm start
This simple setup creates an API Gateway that listens on port 8080. You can now configure it to route requests to the respective microservices.
Step 3: Setting Up the Services with Node.js and Express
Let’s create the User Service first. For this, we’ll need Node.js and Express:
mkdir user-service
cd user-service
npm init -y
npm install express mongoose
Now, create a basic Express server:
const express = require('express');
const mongoose = require('mongoose');
const app = express();
app.use(express.json());
mongoose.connect('mongodb://localhost:27017/users', { useNewUrlParser: true, useUnifiedTopology: true });
const userSchema = new mongoose.Schema({
username: String,
password: String
});
const User = mongoose.model('User', userSchema);
app.post('/register', async (req, res) => {
const user = new User(req.body);
await user.save();
res.send('User registered');
});
app.listen(3001, () => {
console.log('User service running on port 3001');
});
This sets up the user registration service. You can repeat similar steps for Product and Order services.
Step 4: Creating a Centralized Database with MongoDB
Each service can have its own database, but for simplicity, we’ll create one central database using MongoDB to handle all services.
Install MongoDB locally or use a cloud service like MongoDB Atlas. Connect each microservice to this database by replacing the MongoDB URL in each service’s configuration file.
Step 5: Deploying with Docker
We will containerize our services using Docker:
-
Create a
Dockerfile
for each service:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["node", "index.js"]
-
Build and run your Docker containers:
docker build -t user-service .
docker run -p 3001:3001 user-service
Repeat these steps for the other services.
Testing and Scaling the Application
Once your services are running, you can use Postman or cURL to test the endpoints and check if they work together. Scaling is as simple as running more instances of a service. You can do this easily with Docker Compose or Kubernetes.
For example, with Docker Compose, define multiple services in a docker-compose.yml
file and use docker-compose up
to start all services:
version: '3'
services:
user-service:
build: ./user-service
ports:
- "3001:3001"
product-service:
build: ./product-service
ports:
- "3002:3002"
Kubernetes can help you scale further by automating deployment and orchestration, making it easier to handle growing traffic.
Conclusion
Wow, what a journey it’s been! As we finish up this serious plunge into microservices architecture, I hope you’re feeling inspired and equipped to tackle your own projects. Building applications using microservices is about writing code and adopting a mindset that allows you to create systems that can grow and adapt alongside your business needs.
Throughout our time together, we've explored how to design, build, and deploy a microservices application. And while this guide is just a starting point, the world of microservices is vast and full of opportunities. Tools like Kubernetes can take your application to new heights, helping you manage and scale with ease.
If you’re thinking about building a scalable microservices architecture for your business, remember, you don’t have to do it alone. My team and I are here to help you navigate this exciting terrain. Check out our service page to see how we can work together to bring your vision to life. Let’s make something amazing happen!
If you’re looking to build a robust, scalable microservices architecture for your business but need expert guidance, visit my service page to learn how we can help you design, deploy, and maintain enterprise-level microservices systems tailored to your specific needs.
Frequently Asked Questions
What are microservices?
Microservices are a modern architectural style that breaks down applications into smaller, independent services. Each service handles a specific function, making it easier to develop, deploy, and scale applications.
What are the key benefits of microservices architecture?
Microservices architecture offers scalability, fault isolation, and easier deployment. It allows teams to work on different services simultaneously, improving collaboration and speeding up development.
How do I get started with building microservices?
To start building microservices, choose a tech stack like Node.js and MongoDB. Plan your services, set up an API Gateway, and use Docker for deployment. Our guide provides a detailed step-by-step tutorial!
Why should I use Docker for microservices?
Docker simplifies the deployment of microservices by containerizing applications. This ensures consistent environments, easy scalability, and better resource utilization, making it ideal for modern application development.
How can I scale my microservices application?
You can scale your microservices application by deploying additional instances of each service using tools like Docker Compose or Kubernetes. These tools automate scaling, helping you handle increased traffic efficiently.
Share to:
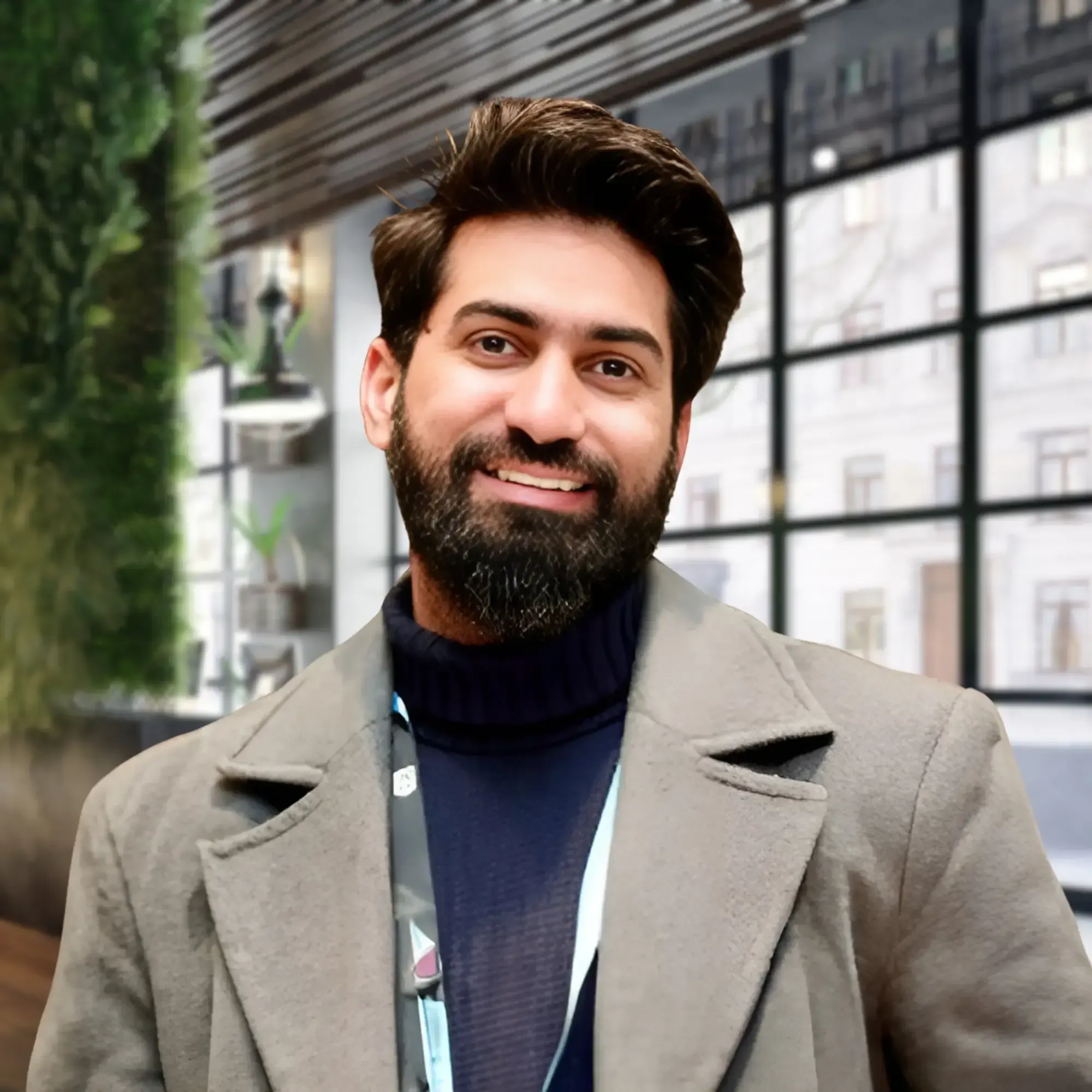
Written By:
Furqan AzizFurqan Aziz is CEO & Founder of InvoZone. He is a tech enthusiast by heart with 10+ years ... Know more
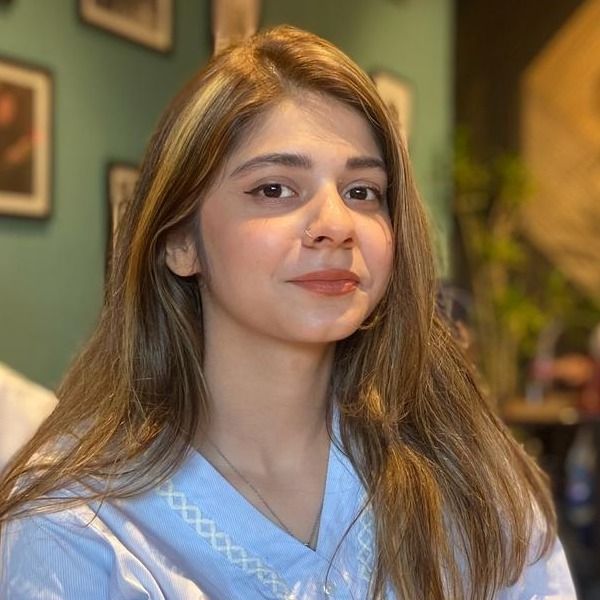
Contributed By:
Senior Content Lead
Get Help From Experts At InvoZone In This Domain