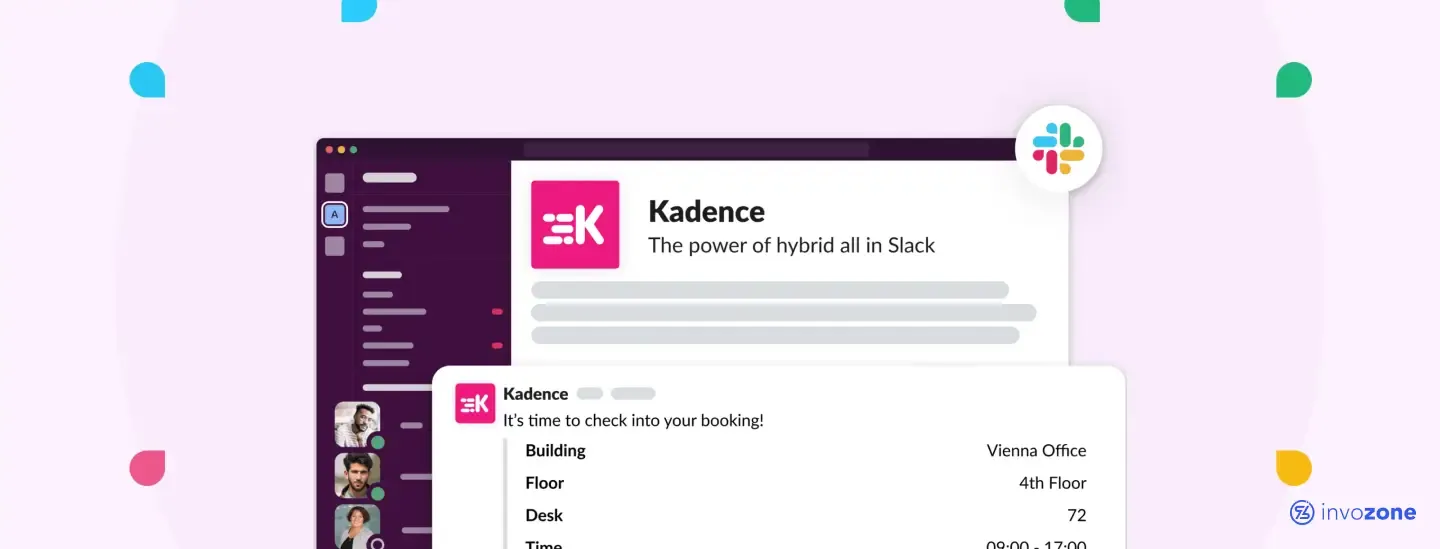
Build a Slack app to proofread messages with ChatGPT API
Discover how to build a Slack app that leverages the ChatGPT API for proofreading messages. This guide walks you through setting up the app, integrating it with Slack, and using a slash command to ensure your communications are clear and error-free. Enhance team professionalism effortlessly.
Published On: 04 September, 2024
4 min read
Table of Contents
Today, ensuring that every message is clear and precise is essential for maintaining effective collaboration. As teams increasingly rely on Slack for their daily interactions, the ability to quickly and accurately proofread messages becomes crucial.
Think about a tool that integrates into your Slack workflow and instantly corrects errors, ensuring that your communications are always polished.
This guide will walk you through the creation of a Slack app that utilizes the ChatGPT API to proofread messages via a simple slash command. By implementing this solution, you will not only streamline your communication process but also provide your team with a tool that guarantees professionalism in every message.
Build Your Slack Proofreading App
- Enhanced Communication Quality: Automatically proofread messages to ensure they are clear, precise, and free of errors.
- Improved Workflow Efficiency: Integrate a powerful AI tool into your existing Slack environment without disrupting your current processes.
- User-Friendly Interaction: Utilize a straightforward slash command to trigger the proofreading functionality, making it accessible and easy to use for all team members.
- Reduced Manual Editing: Save time and reduce the need for manual proofreading by automating the process with advanced AI capabilities.
- Increased Professionalism: Ensure that all written communication meets high standards, reflecting positively on your team's overall professionalism.
Prerequisites
Before starting, ensure you have the following:
- Slack Account: You need a Slack account to create and manage your app.
- Slack App Configuration: Access to the Slack API and the ability to create a new Slack app.
- OpenAI API Key: Sign up at OpenAI and obtain an API key.
- Node.js Installed: Ensure Node.js is installed on your machine.
- Basic Knowledge of JavaScript, Node.js, and Slack API: Familiarity with these technologies will be beneficial.
Step 1: Creating a Slack App
- Create a New Slack App:
- Go to the Slack API and click on "Create New App".
- Choose "From scratch" and give your app a name and workspace to develop in.
- Set Up Slash Command:
- Go to the Slack API and click on "Create New App".
- Choose "From scratch" and give your app a name and workspace to develop in.
- Command: /proofread
- Request URL: This will be the URL of your server that processes the command.
- Short Description: "Proofreads your message."
- Save the settings.
- Add Bot Token Scopes:
- Navigate to "OAuth & Permissions".
- Under "Bot Token Scopes", add the following scopes:
- commands
- chat:write
- Install the app to your workspace, and Slack will provide you with a Bot User OAuth Access Token.
Step 2: Setting Up Your Node.js Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir slack-proofreader
cd slack-proofreader
npm init -y
This initializes a new Node.js project with a package.json file.
Step 3: Install Required Packages
Install the required packages to interact with Slack and OpenAI:
npm install express axios @slack/bolt dotenv
- express: To set up the server.
- axios: To make HTTP requests to the OpenAI API.
- @slack/bolt: To simplify interactions with the Slack API.
- dotenv: To manage environment variables.
Step 4: Set Up Environment Variables
Create a .env file in the root of your project to store your Slack token and OpenAI API key:
SLACK_BOT_TOKEN=your-slack-bot-token
SLACK_SIGNING_SECRET=your-slack-signing-secret
OPENAI_API_KEY=your-openai-api-key
Replace the placeholders with your actual Slack Bot Token, Slack Signing Secret, and OpenAI API key.
Step 5: Writing the Code for Proofreading
Create a new file index.js to handle the logic for proofreading:
- Load Environment Variables and Import Required Modules:
require('dotenv').config();
const { App } = require('@slack/bolt');
const axios = require('axios');
This code loads the environment variables from the .env file and imports the necessary libraries.
-
Initialize the Slack App:
const app = new App({
token: process.env.SLACK_BOT_TOKEN,
signingSecret: process.env.SLACK_SIGNING_SECRET
});
This initializes the Slack app using the provided bot token and signing secret.
-
Set Up the Proofreading Functionality:
Create a function to call the OpenAI API and return a proofread version of the text:
async function proofreadText(text) {
const apiKey = process.env.OPENAI_API_KEY;
try {
const response = await axios.post(
'https://api.openai.com/v1/completions',
{
model: 'text-davinci-003',
prompt: `Proofread the following text: "${text}"`,
max_tokens: 60,
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json',
},
}
);
const proofreadText = response.data.choices[0].text.trim();
return proofreadText;
} catch (error) {
console.error('Error proofreading text:', error.response.data);
return 'Error in proofreading';
}
}
This function interacts with the OpenAI API to proofread the input text and returns the proofread version.
-
Handle Slash Command:
Set up the Slack app to handle the /proofread slash command:
app.command('/proofread', async ({ command, ack, respond }) => {
await ack();
const originalText = command.text;
if (!originalText) {
await respond("Please provide some text to proofread.");
return;
}
const proofreadText = await proofreadText(originalText);
await respond(`*Original:* {originalText}\n*Proofread:* {proofreadText}`);
});
This code does the following:
- Acknowledges the slash command using ack().
- Retrieves the text from the command.
- Calls the proofreadText function to get the proofread text.
- Responds back to the user with the original and proofread text.
-
Start the Server:
Finally, start the server to listen for requests:
(async () => {
await app.start(process.env.PORT || 3000);
console.log('Slack app is running!');
})();
This starts the Slack app server on the specified port.
Step 6: Running and Testing the Application
To start your server and test the proofreading feature:
- Start the Server:
node index.js
-
Test the Slack Command:
Open Slack, type /proofread followed by the text you want to proofread, and press Enter.
Example:
/proofread This is a smaple text with some erros.
The bot should respond with a proofread version of your text:
*Original:* This is a smaple text with some erros.
*Proofread:* This is a sample text with some errors.
Step 7: Areas to Be Careful About
- API Costs: OpenAI charges for API usage, so be mindful of the costs associated with using the ChatGPT API, especially in a production environment.
- Slash Command Limits: Slack enforces rate limits on slash commands, so ensure your app handles potential rate limit errors gracefully.
- Security: Secure your app by validating the Slack signature to ensure requests are genuinely coming from Slack.
How to test this app now?
Testing a Slack app locally involves a few additional steps, as Slack needs to communicate with your local development environment. Here’s how you can set it up:
Step 1: Use a Tunneling Service (e.g., ngrok)
To allow Slack to send requests to your local environment, you'll need to expose your local server to the internet. This can be done using a tunneling service like ngrok.
- Install ngrok:
- Download and install ngrok from ngrok.com.
- Start ngrok:
- In your terminal, start ngrok to forward a public URL to your local server port (e.g., port 3000):
ngrok http 3000
-
- After running this command, ngrok will provide a public URL (e.g., https://12345.ngrok.io) that forwards to your local server at http://localhost:3000.
Step 2: Update Slack App Configuration
- Update Slash Command Request URL:
- Go back to your Slack app's configuration on the Slack API website.
- Navigate to "Slash Commands".
- Update the Request URL for your /proofread command to the ngrok URL provided (e.g., https://12345.ngrok.io/slack/events).
- Reinstall the App:
- After updating the request URL, you might need to reinstall the app in your workspace to ensure the changes take effect.
Step 3: Run Your Local Server
- Start Your Node.js Server:
- Ensure your server is running locally:
node index.js
- Test the Slash Command:
- Open your Slack workspace where the app is installed.
- Type the /proofread slash command followed by some text to test it:
/proofread This is a test message.
-
- Your server should receive the request from Slack via the ngrok tunnel, process it, and send a response back to Slack.
Step 4: Debugging and Logs
- Check ngrok Requests:
- ngrok provides a web interface where you can see the HTTP requests and responses. This is useful for debugging.
- Open http://localhost:4040 in your browser to see the requests.
- Check Server Logs:
- Your Node.js server will log any console messages, including errors, which can help you debug any issues with the app.
Get Started Now And Enhance Your Team’s Communication
Don’t Let Errors Slip Through The Cracks. Build Your Own Slack Proofreading App With Our Step-By-Step Guide
Start NowConclusion
Effective communication is a cornerstone of success in any organization. By developing a Slack app that integrates with the ChatGPT API for proofreading, you have introduced a powerful resource that ensures all written interactions are clear and accurate.
This guide has detailed the necessary steps to create and deploy a Slack app that leverages AI to review and refine messages, addressing a common challenge with a practical solution. As you continue to refine this tool, consider exploring additional features that could further benefit your team.
With ongoing development and innovation, you can ensure that your communication remains precise and impactful, encouraging better collaboration and achieving your organizational goals.
Don’t Have Time To Read Now? Download It For Later.
Table of Contents
Today, ensuring that every message is clear and precise is essential for maintaining effective collaboration. As teams increasingly rely on Slack for their daily interactions, the ability to quickly and accurately proofread messages becomes crucial.
Think about a tool that integrates into your Slack workflow and instantly corrects errors, ensuring that your communications are always polished.
This guide will walk you through the creation of a Slack app that utilizes the ChatGPT API to proofread messages via a simple slash command. By implementing this solution, you will not only streamline your communication process but also provide your team with a tool that guarantees professionalism in every message.
Build Your Slack Proofreading App
- Enhanced Communication Quality: Automatically proofread messages to ensure they are clear, precise, and free of errors.
- Improved Workflow Efficiency: Integrate a powerful AI tool into your existing Slack environment without disrupting your current processes.
- User-Friendly Interaction: Utilize a straightforward slash command to trigger the proofreading functionality, making it accessible and easy to use for all team members.
- Reduced Manual Editing: Save time and reduce the need for manual proofreading by automating the process with advanced AI capabilities.
- Increased Professionalism: Ensure that all written communication meets high standards, reflecting positively on your team's overall professionalism.
Prerequisites
Before starting, ensure you have the following:
- Slack Account: You need a Slack account to create and manage your app.
- Slack App Configuration: Access to the Slack API and the ability to create a new Slack app.
- OpenAI API Key: Sign up at OpenAI and obtain an API key.
- Node.js Installed: Ensure Node.js is installed on your machine.
- Basic Knowledge of JavaScript, Node.js, and Slack API: Familiarity with these technologies will be beneficial.
Step 1: Creating a Slack App
- Create a New Slack App:
- Go to the Slack API and click on "Create New App".
- Choose "From scratch" and give your app a name and workspace to develop in.
- Set Up Slash Command:
- Go to the Slack API and click on "Create New App".
- Choose "From scratch" and give your app a name and workspace to develop in.
- Command: /proofread
- Request URL: This will be the URL of your server that processes the command.
- Short Description: "Proofreads your message."
- Save the settings.
- Add Bot Token Scopes:
- Navigate to "OAuth & Permissions".
- Under "Bot Token Scopes", add the following scopes:
- commands
- chat:write
- Install the app to your workspace, and Slack will provide you with a Bot User OAuth Access Token.
Step 2: Setting Up Your Node.js Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir slack-proofreader
cd slack-proofreader
npm init -y
This initializes a new Node.js project with a package.json file.
Step 3: Install Required Packages
Install the required packages to interact with Slack and OpenAI:
npm install express axios @slack/bolt dotenv
- express: To set up the server.
- axios: To make HTTP requests to the OpenAI API.
- @slack/bolt: To simplify interactions with the Slack API.
- dotenv: To manage environment variables.
Step 4: Set Up Environment Variables
Create a .env file in the root of your project to store your Slack token and OpenAI API key:
SLACK_BOT_TOKEN=your-slack-bot-token
SLACK_SIGNING_SECRET=your-slack-signing-secret
OPENAI_API_KEY=your-openai-api-key
Replace the placeholders with your actual Slack Bot Token, Slack Signing Secret, and OpenAI API key.
Step 5: Writing the Code for Proofreading
Create a new file index.js to handle the logic for proofreading:
- Load Environment Variables and Import Required Modules:
require('dotenv').config();
const { App } = require('@slack/bolt');
const axios = require('axios');
This code loads the environment variables from the .env file and imports the necessary libraries.
-
Initialize the Slack App:
const app = new App({
token: process.env.SLACK_BOT_TOKEN,
signingSecret: process.env.SLACK_SIGNING_SECRET
});
This initializes the Slack app using the provided bot token and signing secret.
-
Set Up the Proofreading Functionality:
Create a function to call the OpenAI API and return a proofread version of the text:
async function proofreadText(text) {
const apiKey = process.env.OPENAI_API_KEY;
try {
const response = await axios.post(
'https://api.openai.com/v1/completions',
{
model: 'text-davinci-003',
prompt: `Proofread the following text: "${text}"`,
max_tokens: 60,
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json',
},
}
);
const proofreadText = response.data.choices[0].text.trim();
return proofreadText;
} catch (error) {
console.error('Error proofreading text:', error.response.data);
return 'Error in proofreading';
}
}
This function interacts with the OpenAI API to proofread the input text and returns the proofread version.
-
Handle Slash Command:
Set up the Slack app to handle the /proofread slash command:
app.command('/proofread', async ({ command, ack, respond }) => {
await ack();
const originalText = command.text;
if (!originalText) {
await respond("Please provide some text to proofread.");
return;
}
const proofreadText = await proofreadText(originalText);
await respond(`*Original:* {originalText}\n*Proofread:* {proofreadText}`);
});
This code does the following:
- Acknowledges the slash command using ack().
- Retrieves the text from the command.
- Calls the proofreadText function to get the proofread text.
- Responds back to the user with the original and proofread text.
-
Start the Server:
Finally, start the server to listen for requests:
(async () => {
await app.start(process.env.PORT || 3000);
console.log('Slack app is running!');
})();
This starts the Slack app server on the specified port.
Step 6: Running and Testing the Application
To start your server and test the proofreading feature:
- Start the Server:
node index.js
-
Test the Slack Command:
Open Slack, type /proofread followed by the text you want to proofread, and press Enter.
Example:
/proofread This is a smaple text with some erros.
The bot should respond with a proofread version of your text:
*Original:* This is a smaple text with some erros.
*Proofread:* This is a sample text with some errors.
Step 7: Areas to Be Careful About
- API Costs: OpenAI charges for API usage, so be mindful of the costs associated with using the ChatGPT API, especially in a production environment.
- Slash Command Limits: Slack enforces rate limits on slash commands, so ensure your app handles potential rate limit errors gracefully.
- Security: Secure your app by validating the Slack signature to ensure requests are genuinely coming from Slack.
How to test this app now?
Testing a Slack app locally involves a few additional steps, as Slack needs to communicate with your local development environment. Here’s how you can set it up:
Step 1: Use a Tunneling Service (e.g., ngrok)
To allow Slack to send requests to your local environment, you'll need to expose your local server to the internet. This can be done using a tunneling service like ngrok.
- Install ngrok:
- Download and install ngrok from ngrok.com.
- Start ngrok:
- In your terminal, start ngrok to forward a public URL to your local server port (e.g., port 3000):
ngrok http 3000
-
- After running this command, ngrok will provide a public URL (e.g., https://12345.ngrok.io) that forwards to your local server at http://localhost:3000.
Step 2: Update Slack App Configuration
- Update Slash Command Request URL:
- Go back to your Slack app's configuration on the Slack API website.
- Navigate to "Slash Commands".
- Update the Request URL for your /proofread command to the ngrok URL provided (e.g., https://12345.ngrok.io/slack/events).
- Reinstall the App:
- After updating the request URL, you might need to reinstall the app in your workspace to ensure the changes take effect.
Step 3: Run Your Local Server
- Start Your Node.js Server:
- Ensure your server is running locally:
node index.js
- Test the Slash Command:
- Open your Slack workspace where the app is installed.
- Type the /proofread slash command followed by some text to test it:
/proofread This is a test message.
-
- Your server should receive the request from Slack via the ngrok tunnel, process it, and send a response back to Slack.
Step 4: Debugging and Logs
- Check ngrok Requests:
- ngrok provides a web interface where you can see the HTTP requests and responses. This is useful for debugging.
- Open http://localhost:4040 in your browser to see the requests.
- Check Server Logs:
- Your Node.js server will log any console messages, including errors, which can help you debug any issues with the app.
Get Started Now And Enhance Your Team’s Communication
Don’t Let Errors Slip Through The Cracks. Build Your Own Slack Proofreading App With Our Step-By-Step Guide
Start NowConclusion
Effective communication is a cornerstone of success in any organization. By developing a Slack app that integrates with the ChatGPT API for proofreading, you have introduced a powerful resource that ensures all written interactions are clear and accurate.
This guide has detailed the necessary steps to create and deploy a Slack app that leverages AI to review and refine messages, addressing a common challenge with a practical solution. As you continue to refine this tool, consider exploring additional features that could further benefit your team.
With ongoing development and innovation, you can ensure that your communication remains precise and impactful, encouraging better collaboration and achieving your organizational goals.
Share to:
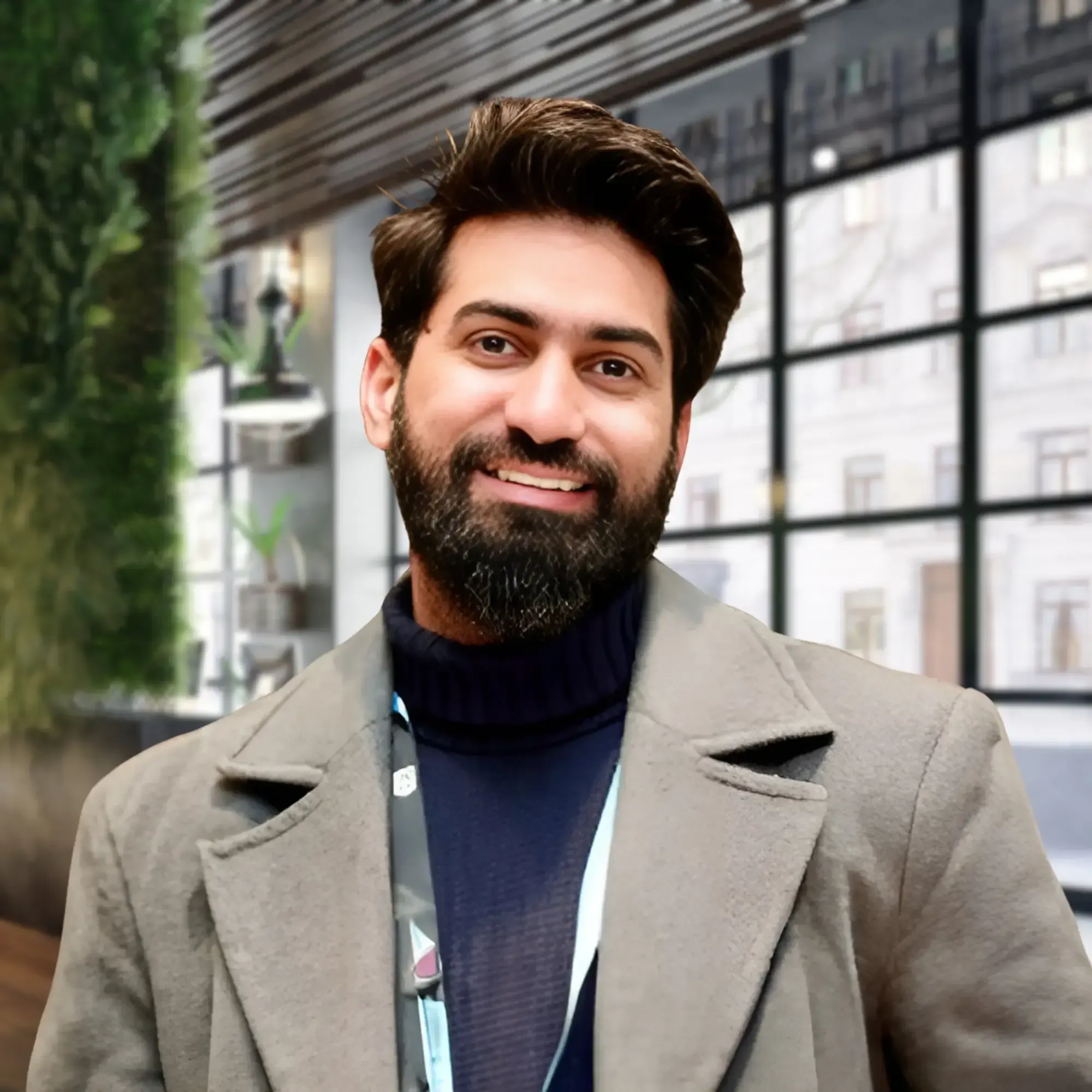
Written By:
Furqan AzizFurqan Aziz is CEO & Founder of InvoZone. He is a tech enthusiast by heart with 10+ years ... Know more
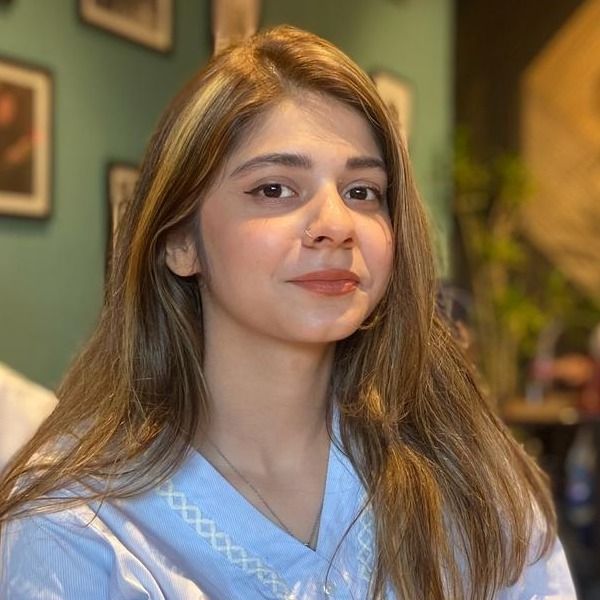
Contributed By:
Senior Content Lead
Get Help From Experts At InvoZone In This Domain